Java Remove All Characters Before Specific One Character
When working with strings in Java, we may encounter scenarios where we need to remove all characters before a particular delimiter or character. Fortunately, we can accomplish this task using various techniques in Java, such as traditional looping, string manipulation methods, or regular expressions. In this tutorial, we’ll explore several approaches to remove all characters before a specified character in a string.
1. Introduction
When working with strings in Java, you may encounter situations where you need to manipulate text to extract a portion of it based on a specific one character. For instance, you might want to remove all characters before a specific one character, such as a colon (:
), a hyphen (-
), or any other delimiter, to isolate the relevant part of the string.
This tutorial will guide you through the process of removing all characters before a specific character in Java. We’ll explore various methods to achieve this, including using String
methods like substring()
and indexOf()
, as well as leveraging regular expressions for more complex scenarios. By the end of this tutorial, you’ll be equipped with the knowledge to handle similar string manipulation tasks in your Java applications efficiently.
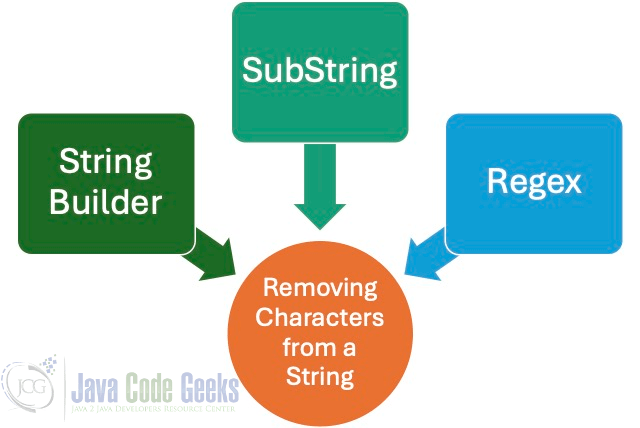
Now let us look at different methods to remove all characters before a specific character.
2. Remove All Characters Before a Specific Character Using Indexing and Substrings
In Java, a substring is a portion of a string extracted from a larger string. The String class provides several methods to work with substrings, with the most commonly used being the substring() method. This method allows you to extract a specific part of a string based on index positions.
Java Code for the program to Remove All Characters Before a Specific Character :
Let us look at the java code for the implementation:
public class RemoveCharactersSubStringExample { public static void main(String[] args) { String inputString = "This is an example"; char targetCharacters = 'n'; int index = inputString.indexOf(targetCharacters); if (index != -1) { String result = inputString.substring(index); System.out.println(result); } else { System.out.println(inputString); } } }
You can compile and execute the above code with the following commands:
javac RemoveCharactersSubStringExample.java java RemoveCharactersSubStringExample
The output for the above commands when executed is shown below:
bhagvanarch@Bhagvans-MacBook-Air remove_characters_aug_31 % java RemoveCharactersSubStringExample n example bhagvanarch@Bhagvans-MacBook-Air remove_characters_aug_31 %
PseudoCode for Two-step Algorithm to Remove All Characters Before a Specific Character
1. Find the Index: Use indexOf(targetCharacter)
to find the position of the target character in the string.
2. Check Existence: Ensure the character exists in the string (index != -1
).
3. Extract Substring: Use substring(index)
to get the part of the string starting from the target character.
This method is efficient and straightforward
Example Usage:
For a string "This is an example" and character 'n'
, this code will print:
n example
3. Remove All Characters Before a Specific Character Using Regular Expressions
Regular expressions, commonly known as regex, are a powerful tool for pattern matching and text manipulation. In Java, regular expressions are handled by the java.util.regex package, which provides classes such as Pattern and Matcher to work with regex.
Basic Concepts of Regular Expressions
• Pattern: A sequence of characters that defines a search pattern. This pattern can be used to match, locate, and manage text.
• Matcher: An engine that performs match operations on a character sequence by interpreting a Pattern.
Java Code for the program to Remove All Characters Before a Specific Character:
Let us look at the java code for the implementation:
public class RemoveCharactersExample { public static void main(String[] args) { String inputString = "This is an example"; char targetCharacters = 'n'; String regex = ".*" + targetCharacters; String result = inputString.replaceAll(regex, String.valueOf(targetCharacters)); System.out.println(result); } }
You can compile and execute the above code with the following commands:
javac RemoveCharactersExample.java java RemoveCharactersExample
The output for the above commands when executed is shown below:
bhagvanarch@Bhagvans-MacBook-Air remove_characters_aug_31 % java RemoveCharactersExample n example bhagvanarch@Bhagvans-MacBook-Air remove_characters_aug_31 %
PseudoCode for Two-step Algorithm to Remove All Characters Before a Specific Character:
1. Regex Pattern: The pattern ".*" + targetCharacter
matches any sequence of characters (.*
) followed by the target character.
2. Replace All: The replaceAll()
method replaces the matched sequence with the target character itself, effectively removing all characters before it.
This method is powerful and concise,
Example Usage:
For a string "This is an example" and character 'n'
, this code will print:
n example
4. Remove All Characters Before a Specific Character Using StringBuilder
The StringBuilder class in Java is used to create mutable sequences of characters. Unlike String, which is immutable (meaning once a String object is created, it cannot be modified), StringBuilder allows you to modify the content without creating new objects, making it more efficient for operations that involve frequent changes to the string content.
Key Features of StringBuilder
• Mutability: Unlike String, which creates a new object every time you modify it, StringBuilder allows you to change the content of the same object.
• Efficient Memory Usage: Since StringBuilder doesn’t create new objects with every modification, it can be more memory-efficient, especially in loops or when concatenating large strings.
• Not Thread-Safe: StringBuilder is not synchronized, meaning it is not thread-safe. If you need a thread-safe version, you should use StringBuffer, which is synchronized but slightly slower.
Remove All Characters Before a Specific Character Using StringBuilder
Let us look at the java code for the implementation:
public class RemoveCharactersStringBuilderExample { public static void main(String[] args) { String inputString = "This is an example"; char targetCharacters = 'n'; StringBuilder sb = new StringBuilder(inputString); int index = sb.indexOf(String.valueOf(targetCharacters)); if (index != -1) { sb.delete(0, index); System.out.println(sb.toString()); } else { System.out.println("Character not found in the string."); } } }
You can compile and execute the above code with the following commands:
javac RemoveCharactersStringBuilderExample.java java RemoveCharactersStringBuilderExample
The output for the above commands when executed is shown below:
bhagvanarch@Bhagvans-MacBook-Air remove_characters_aug_31 % java RemoveCharactersStringBuilderExample n example bhagvanarch@Bhagvans-MacBook-Air remove_characters_aug_31 %
Example Usage:
For a string "This is an example" and character 'n'
, this code will print:
n example
5. Conclusion
In this tutorial, we explored various methods to remove all characters before a specific character in a Java string. Here’s a quick recap:
- Using
substring()
:- Efficiently extracts the part of the string starting from the target character.Example:
inputString.substring(index)
.
- Efficiently extracts the part of the string starting from the target character.Example:
- Using Regular Expressions:
- Utilizes regex patterns to match and replace characters.
- Example:
inputString.replaceAll(".*" + targetCharacter, String.valueOf(targetCharacter))
.
- Using
StringBuilder
:- Leverages
StringBuilder
for flexible and efficient string manipulation.Example:sb.delete(0, index)
.
- Leverages
Each method has its advantages:
substring()
is straightforward and easy to use.- Regular Expressions offer powerful pattern-matching capabilities.
StringBuilder
provides efficient and mutable string operations.
By understanding these techniques, you can choose the most suitable approach for your specific needs. Happy coding!
If you have any questions or need further assistance, feel free to ask!.
6. Download
You can download the full source code of this example here: Remove All Characters Before a Specific Character in Java