Read replicas and Spring Data Part 3: Configuring two entity managers
Our previous setup works as expected. What we shall do now is to get one step further and configure two separate entity managers without affecting the functionality we achieved previously.
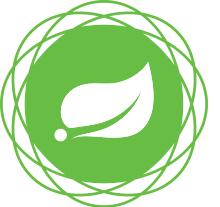
The first step would be to set the default entity manager configuration to a primary one.
This is the first step
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 | package com.gkatzioura.springdatareadreplica.config; import javax.sql.DataSource; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.beans.factory.annotation.Value; import org.springframework.boot.jdbc.DataSourceBuilder; import org.springframework.boot.orm.jpa.EntityManagerFactoryBuilder; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.Primary; import org.springframework.data.jpa.repository.config.EnableJpaRepositories; import org.springframework.orm.jpa.LocalContainerEntityManagerFactoryBean; @Configuration public class PrimaryEntityManagerConfiguration { @Value ( "${spring.datasource.username}" ) private String username; @Value ( "${spring.datasource.password}" ) private String password; @Value ( "${spring.datasource.url}" ) private String url; @Bean @Primary public DataSource dataSource() throws Exception { return DataSourceBuilder.create() .url(url) .username(username) .password(password) .driverClassName( "org.postgresql.Driver" ) .build(); } @Bean @Primary public LocalContainerEntityManagerFactoryBean entityManagerFactory( EntityManagerFactoryBuilder builder, @Qualifier ( "dataSource" ) DataSource dataSource) { return builder.dataSource(dataSource) .packages( "com.gkatzioura.springdatareadreplica" ) .persistenceUnit( "main" ) .build(); } } |
If you run your application with this configuration it will run just like our application previously.
Now it is time to configure the read only entity manager.
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 | package com.gkatzioura.springdatareadreplica.config; import javax.sql.DataSource; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.beans.factory.annotation.Value; import org.springframework.boot.jdbc.DataSourceBuilder; import org.springframework.boot.orm.jpa.EntityManagerFactoryBuilder; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Primary; import org.springframework.orm.jpa.LocalContainerEntityManagerFactoryBean; @Configuration public class ReadOnlyEntityManagerConfiguration { @Value ( "${spring.datasource.username}" ) private String username; @Value ( "${spring.datasource.password}" ) private String password; @Value ( "${spring.datasource.readUrl}" ) private String readUrl; @Bean public DataSource readDataSource() throws Exception { return DataSourceBuilder.create() .url(readUrl) .username(username) .password(password) .driverClassName( "org.postgresql.Driver" ) .build(); } @Bean public LocalContainerEntityManagerFactoryBean readEntityManagerFactory( EntityManagerFactoryBuilder builder, @Qualifier ( "readDataSource" ) DataSource dataSource) { return builder.dataSource(dataSource) .packages( "com.gkatzioura.springdatareadreplica" ) .persistenceUnit( "read" ) .build(); } } |
Also I will add a method to a controller in order to save the models.
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | package com.gkatzioura.springdatareadreplica.controller; import java.util.List; import org.springframework.http.HttpStatus; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.ResponseStatus; import org.springframework.web.bind.annotation.RestController; import com.gkatzioura.springdatareadreplica.entity.Employee; import com.gkatzioura.springdatareadreplica.repository.EmployeeRepository; @RestController public class EmployeeContoller { private final EmployeeRepository employeeRepository; public EmployeeContoller(EmployeeRepository employeeRepository) { this .employeeRepository = employeeRepository; } @GetMapping ( "/employee" ) public List<Employee> getEmployees() { return employeeRepository.findAll(); } @PostMapping ( "/employee" ) @ResponseStatus (HttpStatus.CREATED) public void addEmployee( @RequestBody Employee employee) { employeeRepository.save(employee); } } |
If you do try to add the an employee using the controller and then query the read database you shall see that no entry is being added at all.
So we have our primary entity manager up and running and we also have a secondary one. The secondary one is not used yet. The next blog focuses on putting the secondary read only entity manager in use.
Published on Java Code Geeks with permission by Emmanouil Gkatziouras, partner at our JCG program. See the original article here: Read replicas and Spring Data Part 3: Configuring two entity managers Opinions expressed by Java Code Geeks contributors are their own. |