Spring Boot and SOLID: A Perfect Match
Spring Boot, a popular Java framework for building microservices and web applications, has gained immense popularity due to its simplicity, convention over configuration approach, and rapid development capabilities. However, to create robust, maintainable, and scalable applications, it’s essential to adhere to sound design principles. The SOLID principles, a set of five object-oriented design principles, provide a framework for writing clean, modular, and extensible code.
In this article, we will explore how Spring Boot can be used to effectively implement the SOLID principles. We will delve into each principle, discuss its benefits, and provide practical examples using Spring Boot. By understanding and applying these principles, developers can create Spring Boot applications that are not only efficient but also easy to maintain and extend over time.
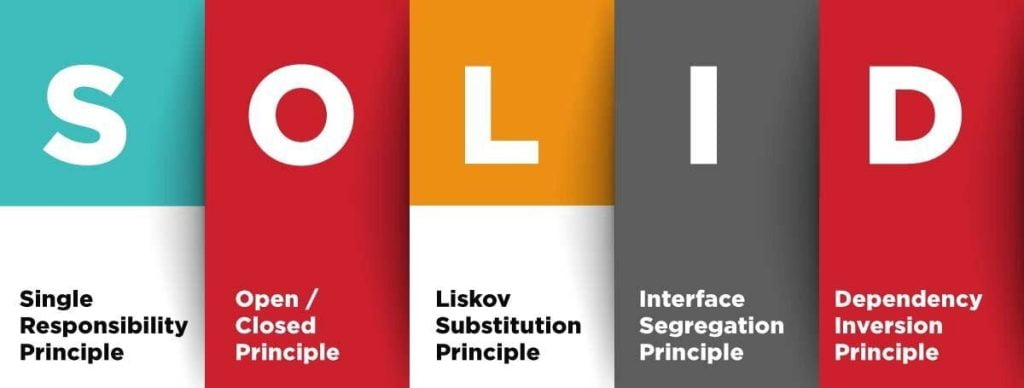
1. Understanding SOLID Principles
Single Responsibility Principle (SRP)
Definition: A class should have only one reason to change. In other words, a class should have a single responsibility.
Benefits:
- Maintainability: Classes that adhere to SRP are easier to understand, modify, and test.
- Flexibility: Classes with a single responsibility are more reusable and can be adapted to changing requirements without affecting other parts of the system.
- Testability: Classes with a single responsibility are easier to test, as they have a clear and focused purpose.
Spring Boot Example: Consider a UserService
class in a Spring Boot application. Instead of having methods for user registration, authentication, and authorization, it could be broken down into separate classes: UserRegistrationService
, AuthenticationService
, and AuthorizationService
. This adheres to SRP by assigning each class a specific responsibility.
@Service public class UserRegistrationService { @Autowired private UserRepository userRepository; public User registerUser(UserRegistrationRequest request) { // ... registration logic } } @Service public class AuthenticationService { @Autowired private UserRepository userRepository; public boolean authenticateUser(AuthenticationRequest request) { // ... authentication logic } } @Service public class AuthorizationService { @Autowired private UserRepository userRepository; public boolean isUserAuthorized(String username, String role) { // ... authorization logic } }
Open-Closed Principle (OCP)
Definition: Entities should be open for extension but closed for modification. This means that you can add new functionality to a class without modifying its existing code.
Benefits:
- Extensibility: OCP allows you to add new features to your application without breaking existing code.
- Maintainability: By avoiding modifications to existing code, OCP reduces the risk of introducing bugs.
- Flexibility: OCP makes your application more adaptable to changing requirements.
Spring Boot Example: Suppose you have a NotificationService
class that sends notifications via email. To add support for SMS notifications, you can create a new SmsNotificationService
class that implements the same interface as EmailNotificationService
. This allows you to extend the functionality of your application without modifying the existing NotificationService
class.
public interface NotificationService { void sendNotification(NotificationMessage message); } @Service public class EmailNotificationService implements NotificationService { @Autowired private JavaMailSender javaMailSender; @Override public void sendNotification(NotificationMessage message) { // ... send email notification } } @Service public class SmsNotificationService implements NotificationService { @Autowired private SmsService smsService; @Override public void sendNotification(NotificationMessage message) { // ... send SMS notification } }
Liskov Substitution Principle (LSP)
Definition: Objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
Benefits:
- Polymorphism: LSP enables polymorphism, which makes code more flexible and reusable.
- Maintainability: By ensuring that subclasses can be used interchangeably with their superclasses, LSP makes it easier to maintain and extend your application.
- Testability: LSP simplifies testing, as you can write tests against the superclass and be confident that they will also work with its subclasses.
Spring Boot Example: Consider a Shape
interface with methods like calculateArea()
and calculatePerimeter()
. A Rectangle
and Circle
class can implement this interface. According to LSP, any code that expects a Shape
object should be able to use either a Rectangle
or a Circle
without breaking the code.
public interface Shape { double calculateArea(); double calculatePerimeter(); } public class Rectangle implements Shape { private double length; private double width; // ... implementation } public class Circle implements Shape { private double radius; // ... implementation }
Interface Segregation Principle (ISP)
Definition: Clients should not be forced to depend on interfaces they do not use.
Benefits:
- Decoupling: ISP promotes loose coupling between classes, making your application more modular and easier to maintain.
- Testability: ISP simplifies testing by reducing the number of dependencies that need to be mocked.
- Flexibility: ISP makes your application more flexible by allowing you to change the implementation of an interface without affecting its clients.
Spring Boot Example: Instead of having a single UserService
interface with methods for registration, authentication, and authorization, you could create separate interfaces for each responsibility. This allows clients to only depend on the interfaces they need, reducing coupling and improving flexibility.
public interface UserRegistrationService { User registerUser(UserRegistrationRequest request); } public interface AuthenticationService { boolean authenticateUser(AuthenticationRequest request); } public interface AuthorizationService { boolean isUserAuthorized(String username, String role); }
Dependency Inversion Principle (DIP)
Definition: High-level modules should not depend on low-level modules. Both should depend on abstractions. Abstractions should not depend on details. Details should depend on abstractions.
Benefits:
- Decoupling: DIP promotes loose coupling between modules, making your application more modular and easier to maintain.
- Testability: DIP simplifies testing by allowing you to mock dependencies and isolate units of code.
- Flexibility: DIP makes your application more flexible by allowing you to change the implementation of low-level modules without affecting high-level modules.
Spring Boot Example: In Spring Boot, you can use dependency injection to implement DIP. Instead of directly creating instances of low-level classes, you can inject them as dependencies into high-level classes. This allows you to easily replace implementations without modifying the high-level code.
@Service public class UserService { @Autowired private UserRepository userRepository; @Autowired private PasswordEncoder passwordEncoder; public User registerUser(UserRegistrationRequest request) { // ... registration logic using userRepository and passwordEncoder } }
In this example, UserService
depends on the UserRepository
and PasswordEncoder
interfaces, not on their concrete implementations. This allows you to easily replace the implementations of these classes without affecting the UserService
.
2. Applying SOLID Principles in Spring Boot
Spring Boot’s design philosophy aligns well with the SOLID principles, making it an ideal framework for building robust and maintainable applications. Let’s explore how Spring Boot’s features and conventions support SOLID principles and provide practical tips for applying them in your projects.
Alignment of Spring Boot with SOLID Principles
- Single Responsibility Principle (SRP): Spring Boot promotes the creation of small, focused components, such as services and repositories, which naturally adhere to SRP.
- Open-Closed Principle (OCP): Spring Boot’s dependency injection mechanism allows you to easily extend functionality by injecting new components without modifying existing code.
- Liskov Substitution Principle (LSP): Spring Boot’s support for polymorphism and inheritance ensures that subclasses can be used interchangeably with their superclasses.
- Interface Segregation Principle (ISP): Spring Boot’s annotation-based configuration and dependency injection make it easy to define and use fine-grained interfaces, promoting ISP.
- Dependency Inversion Principle (DIP): Spring Boot’s dependency injection framework encourages loose coupling between components, adhering to DIP.
Practical Tips for Applying SOLID Principles
- Break down large components into smaller ones: Divide your application into smaller, focused components to improve maintainability and testability.
- Use interfaces and abstract classes: Define interfaces or abstract classes to promote loose coupling and enable polymorphism.
- Leverage dependency injection: Use Spring Boot’s dependency injection to inject dependencies into your components, promoting loose coupling and testability.
- Avoid god objects: Avoid creating large, monolithic classes that handle multiple responsibilities.
- Use design patterns: Consider using design patterns like Strategy, Template Method, and Factory to implement SOLID principles effectively.
- Write unit tests: Write comprehensive unit tests to ensure that your code adheres to SOLID principles and is easy to maintain.
Common Pitfalls and Anti-Patterns
- God objects: Avoid creating large, monolithic classes that handle multiple responsibilities.
- Tight coupling: Be cautious of tightly coupled components, as it can make your application difficult to maintain and extend.
- Overusing inheritance: Avoid excessive inheritance, as it can make your code complex and hard to understand.
- Ignoring SOLID principles: Don’t neglect SOLID principles in favor of quick development or short-term gains.
- Not using dependency injection: Avoid creating instances of dependencies directly within your components. Use dependency injection to promote loose coupling.
3. Case Study: A Spring Boot Application
Application Overview: Let’s consider a simple e-commerce application that allows users to browse products, add them to a cart, and place orders.
SOLID Principles Implementation:
- Single Responsibility Principle (SRP):
- Product Service: Handles product-related operations like fetching products, searching, and filtering.
- Cart Service: Manages shopping carts, including adding, removing, and updating items.
- OrderService: Processes orders, calculates totals, and updates inventory.
- Open-Closed Principle (OCP):
- PaymentStrategy: Defines an interface for different payment methods (e.g., credit card, PayPal).
- CreditCardPaymentStrategy: Implements the
PaymentStrategy
interface for credit card payments. - PayPalPaymentStrategy: Implements the
PaymentStrategy
interface for PayPal payments.
- Liskov Substitution Principle (LSP):
Product
class can be extended toPhysicalProduct
andDigitalProduct
without breaking existing code.- Both
PhysicalProduct
andDigitalProduct
can be used interchangeably in theCartService
andOrderService
.
- Interface Segregation Principle (ISP):
- Instead of a single
ProductService
interface, we can define separate interfaces for different product-related operations (e.g.,ProductSearchService
,ProductFilteringService
).
- Instead of a single
- Dependency Inversion Principle (DIP):
- Using dependency injection, the
OrderService
can be injected with instances ofPaymentStrategy
,ProductService
, andCartService
, promoting loose coupling.
- Using dependency injection, the
Code Example (Simplified):
@Service public class ProductService { // ... product-related methods } @Service public class CartService { @Autowired private ProductService productService; // ... cart-related methods } @Service public class OrderService { @Autowired private ProductService productService; @Autowired private CartService cartService; @Autowired private PaymentStrategy paymentStrategy; public void placeOrder(Order order) { // ... order processing logic using paymentStrategy, productService, and cartService } } public interface PaymentStrategy { void processPayment(Order order); } @Component public class CreditCardPaymentStrategy implements PaymentStrategy { // ... credit card payment logic } @Component public class PayPalPaymentStrategy implements PaymentStrategy { // ... PayPal payment logic }
Benefits of Using SOLID Principles in the E-commerce Application
Benefit | Explanation |
---|---|
Maintainability | The application is easier to understand, modify, and extend due to the clear separation of concerns. |
Flexibility | New payment methods can be added by simply creating a new PaymentStrategy implementation without modifying existing code. |
Testability | Each component can be tested independently, improving code quality and reducing the risk of bugs. |
Extensibility | New features can be added without affecting existing code, making the application more adaptable to changing requirements. |
Reusability | Components like ProductService and CartService can be reused in other applications. |
4. Wrapping Up
By applying SOLID principles in Spring Boot applications, developers can create more robust, maintainable, and flexible software. The case study demonstrated how these principles can be effectively implemented to build a well-structured e-commerce application. By following SOLID principles, you can improve code quality, enhance flexibility, and simplify testing.