Spring Boot: From Zero to Launch
Have you ever dreamt of building a robust Java application without getting bogged down in complex configurations? Spring Boot makes that dream a reality! This framework empowers you to develop microservices, web applications, and even batch processing tasks with incredible ease and speed.
This guide will serve as your launchpad into the exciting world of Spring Boot. In just a few minutes, you’ll gain the knowledge to:
- Set up a brand new Spring Boot application from scratch.
- Understand the core concepts behind Spring Boot’s streamlined approach.
- Launch your application and witness it spring to life in seconds.
So, grab your favorite beverage, buckle up, and get ready to experience the magic of Spring Boot!
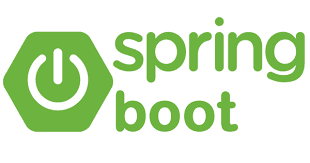
1. Setting Up Your Development Environment
Here’s how to create a new Spring Boot application using Spring Initializr, a web-based tool that simplifies the setup process:
- Visit Spring Initializr: Open your web browser and navigate to https://start.spring.io/.
- Choose Project Details:
- Project Name: Enter a descriptive name for your project (e.g., “spring-boot-intro”). This name will be used for the generated project directory.
- Language: Select “Java” (assuming you’re working with Java development).
- Dependencies: This is where you choose the functionalities you want your application to have. Here are some key choices with explanations:
- Spring Web: This dependency is essential for building web applications with Spring Boot. It allows you to handle HTTP requests and responses.
- Thymeleaf (Optional): This template engine can be used to create dynamic web pages with HTML and server-side logic. It’s optional for this basic example but can be added later for more advanced features.
- Generate Project: Once you’ve selected your desired options, click the “Generate” button. This will download a ZIP file containing your new Spring Boot application project.
- Extract the Project: Extract the downloaded ZIP file to a convenient location on your computer. This will create a new folder with your chosen project name (e.g., “spring-boot-intro”).
Explanation of Key Choices:
- Project Name: This helps identify your project and organize your files. Choose a name that reflects the application’s purpose.
- Spring Web: This dependency provides the foundation for building web applications using Spring Boot. It includes features like handling HTTP requests, routing, and handling responses.
- Thymeleaf (Optional): While not required for this basic example, Thymeleaf offers a way to create dynamic web pages. It allows you to embed server-side logic (like retrieving data from a database) within your HTML templates.
2. Understanding the Spring Boot Project Structure
Spring Boot enforces a well-defined project structure to keep your code organized and maintainable. Here’s a breakdown of the main folders and files you’ll encounter:
1. src/main/java: This folder holds all your application’s core Java source code. Here are some important subfolders:
- com.(your.company.package): This is your main package namespace, typically following your company’s domain or project name.
- Example: If your project is called “spring-boot-intro,” your main package might be
com.example.springbootexample
.
- Example: If your project is called “spring-boot-intro,” your main package might be
- (Your application classes): Inside your package, you’ll find the Java classes that make up your application logic. These might include:
- A class annotated with
@SpringBootApplication
(the main entry point for your application). - Controller classes handling incoming requests (explained later).
- Service classes encapsulating business logic.
- Entity classes representing data models (used for databases or other data persistence).
- A class annotated with
2. src/main/resources: This folder contains non-Java resources needed by your application at runtime. Some key files here include:
- application.properties: This file acts as a central configuration point for your application. You can define various settings like database connection details, logging preferences, or server port configuration.
- static: This subfolder can hold static content like images, CSS stylesheets, or JavaScript files used by your application’s user interface (if applicable).
3. pom.xml (Optional): This file is used for project dependency management with Maven (a build tool). It specifies the external libraries your application relies on, including Spring Boot itself and any other required dependencies chosen during project setup.
This is a simplified overview. Spring Boot applications can incorporate additional folders and files depending on your specific needs and chosen functionalities.
3. Creating a Simple Spring Boot Application (The Core)
Here’s a breakdown of the code for a basic Spring Boot application:
1. Spring Boot Application Class (main.java):
@SpringBootApplication public class SpringBootIntroApplication { public static void main(String[] args) { SpringApplication.run(SpringBootIntroApplication.class, args); } }
- @SpringBootApplication: This annotation marks this class as the main entry point for your Spring Boot application. It automatically enables features like component scanning and auto-configuration, simplifying development.
- public static void main(String[] args): This is the main method where your application execution starts.
SpringApplication.run(SpringBootIntroApplication.class, args);
: This line starts the Spring Boot application using the provided class (SpringBootIntroApplication) and any command-line arguments passed during execution.
2. Simple Controller Class (e.g., HelloWorldController.java):
@RestController // This denotes a REST API controller public class HelloWorldController { @GetMapping("/") // Handles GET requests to the root path ("/") public String helloWorld() { return "Hello World!"; } }
- @RestController: This annotation marks the class as a REST API controller. It combines functionality from
@Controller
(handling requests) and@ResponseBody
(returning data directly in the response body). - @GetMapping(“/”): This annotation defines a request mapping. It specifies that the
helloWorld
method will handle GET requests to the root path of your application (usually “/”).
Explanation:
- This code defines two classes:
SpringBootIntroApplication
: This class serves as the main entry point with the@SpringBootApplication
annotation.HelloWorldController
: This controller class defines a single methodhelloWorld
annotated with@GetMapping("/").
This method will be invoked whenever a GET request is made to the root path of your application. The method simply returns the string “Hello World!” which will be included in the response sent back to the client.
By running this application with mvn spring-boot:run
(assuming you have Maven set up), you’ll create a server instance. If you then make a GET request to http://localhost:8080/
(default port for Spring Boot applications), you should receive the response “Hello World!” indicating your application is running successfully.
4. Running Your First Spring Boot Application (The Magic!)
There are two main ways to run your Spring Boot application:
1. Running from an IDE (Integrated Development Environment):
Most popular IDEs like IntelliJ IDEA, Eclipse, or Spring Tool Suite provide built-in functionality for running Spring Boot applications. Here’s a general process:
- Open your project in your chosen IDE.
- Locate the main application class (usually the one with
@SpringBootApplication
). - Right-click on the class and select the “Run” option. Your IDE will typically handle the necessary steps to launch the application.
- View the application output in the IDE’s console window. This will display logs and messages as your application runs.
2. Running from the Command Line:
For more granular control, you can run your application directly from the command line:
- Open a terminal or command prompt.
- Navigate to the directory containing your project’s pom.xml file. (assuming you’re using Maven for project management)
- Execute the following command:
mvn spring-boot:run
This command instructs Maven to build and run your Spring Boot application. The application output will be displayed in the terminal window.
Hot Reloading for Faster Development:
Spring Boot offers a powerful feature called hot reloading, which significantly streamlines your development workflow. Here’s how it works:
- When you make changes to your Java source code (e.g., modifying the
helloWorld
method in the previous example), Spring Boot automatically detects these changes without the need for a full application restart. - The modified code is recompiled and injected into the running application on-the-fly.
- This allows you to see the effects of your code changes almost instantly, greatly reducing development time and improving iteration speed.
5. Additional Resources:
- Spring Boot Running an Application: https://spring.io/guides/gs/spring-boot
- Spring Boot Hot Reloading: https://www.codejava.net/frameworks/spring-boot/spring-boot-automatic-restart-using-spring-boot-devtools
6. Conclusion
Congratulations! You’ve successfully navigated your way through creating and running your first Spring Boot application. In just few minutes, you’ve learned the fundamentals of setting up a project, understanding core components, and launching your application to experience the magic of Spring Boot.
This is just the beginning of your exciting Spring Boot journey. The framework empowers you to build robust, scalable, and microservice-oriented applications with incredible ease. As you explore further, you’ll discover features like dependency injection, database integration, security configurations, and much more.