Java Array Minimum position – Finding the min element
Operations on arrays are essential, and we might need them in any application. Sometimes, they’re hidden behind more convenient interfaces like Collections API. However, this is the basic knowledge we should acquire early in our careers. In this tutorial, we’ll learn how to find in java array, the minimum element position. We’ll discuss the methods to do so regardless of the types of the elements, but for simplicity, we’ll use an array of integers. In Java arrays, finding the minimum position of the smallest element is a common scenario that every java developer codes.
1. Overview
To find the position of the minimum element in an array, you can use a simple algorithm. Here are the steps for the algorithm :
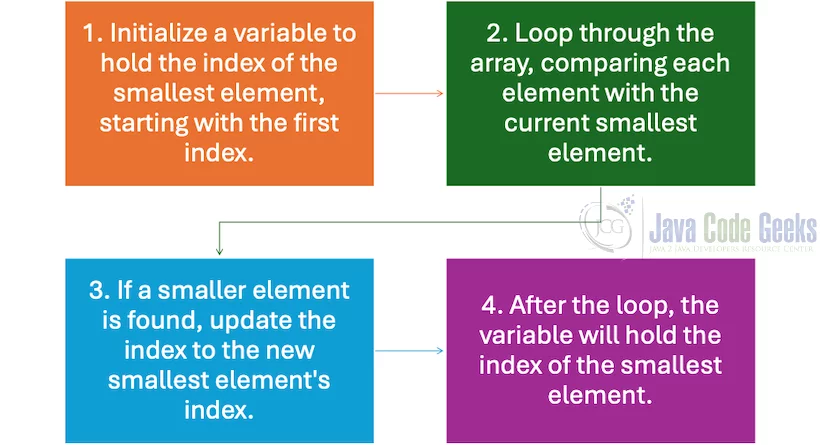
Finding the Index of the smallest element in an array
Now let us look at the simple iteration method implementation of how to find in java array, the minimum element position.
2. Simple Iteration method to find in Java array, the minimum element position.
You can find the index of the smallest element in an array using a simple iterative method in Java. The idea is to loop through the array, keeping track of the smallest element and its index as you go.
Here’s a step-by-step explanation and the corresponding Java code:
Steps for the program to find the minimum element position in Java array:
- Initialize variables: Start by initializing a variable to store the index of the smallest element, initially assuming it’s the first element (index 0).
- Iterate through the array: Use a
for
loop to iterate through the array from the second element (index 1) to the end. - Compare elements: If the current element is smaller than the element at the stored index, update the smallest element index.
- Return the index: After the loop, the variable will contain the index of the smallest element.
Java Code for the program to find the minimum element position in Java array:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | public class LowestElementExample { public int findIndexOfLowestElement( int [] arr) { if (arr == null || arr.length == 0 ) { throw new IllegalArgumentException( "Array must not be null or empty" ); } int minIndex = 0 ; for ( int i = 1 ; i < arr.length; i++) { if (arr[i] < arr[minIndex]) { minIndex = i; } } return minIndex; } public static void main(String[] args) { LowestElementExample example = new LowestElementExample(); int [] test_array = { 7 , 1 , 3 , 1 , 5 , 9 , 8 , 6 }; int minIndex = example.findIndexOfLowestElement(test_array); System.out.println( "Index of the lowest value element: " + minIndex + " Value of the lowest value is :" + test_array[minIndex]); } } |
You can compile and execute the above code with the following commands:
1 2 | javac LowestElementExample.java java LowestElementExample |
The output for the above commands when executed is shown below:
1 2 3 4 | bhagvanarch@Bhagvans-MacBook-Air index_lowest_18_Aug % javac LowestElementExample.java bhagvanarch@Bhagvans-MacBook-Air index_lowest_18_Aug % java LowestElementExample Index of the lowest value element: 1 Value of the lowest value is :1 bhagvanarch@Bhagvans-MacBook-Air index_lowest_18_Aug % |
Code Explanation for the program to find the minimum element position in Java array:
- Input Validation: The method checks if the array is
null
or empty and throws an exception if it is. minIndex
Initialization: TheminIndex
is initialized to0
, assuming the first element is the smallest.for
Loop: The loop starts from index1
and compares each element with the current smallest element. If a smaller element is found,minIndex
is updated to the current index.- Output: After the loop,
minIndex
contains the index of the smallest element.
Example Usage:
For the array {7, 1, 3, 1, 5, 9, 8, 6}
, this code will print:
1 | Index of the lowest value element: 1 Value of the lowest value is :1 |
This method efficiently finds the index of the smallest element in O(n)
time complexity, where n
is the length of the array. This method helps to find in Java array, the minimum element position.
3. Two-Step Approach to find in Java array, the minimum element position
To find the index of the smallest element in an array using a two-step method in Java, you can break down the process into two parts:
- First step: Find the smallest element in the array.
- Second step: Find the index of that smallest element.
This approach may not be as efficient as doing it in a single step, but it separates the concerns of identifying the smallest value and then locating its index. It helps to find in Java array, the minimum element position.
Java Code for the program to find the minimum element position in Java array:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 | public class LowestElementTwoStepExample { public int findLowestElement( int [] arr) { if (arr == null || arr.length == 0 ) { throw new IllegalArgumentException( "Array must not be null or empty" ); } int minElement = arr[ 0 ]; for ( int i = 1 ; i < arr.length; i++) { if (arr[i] < minElement) { minElement = arr[i]; } } return minElement; } public int findIndexOfElement( int [] arr, int element) { for ( int i = 0 ; i < arr.length; i++) { if (arr[i] == element) { return i; } } return - 1 ; } public static void main(String[] args) { int [] array = { 3 , 1 , 4 , 1 , 5 , 9 , 2 , 6 }; LowestElementTwoStepExample example = new LowestElementTwoStepExample(); int lowestElement = example.findLowestElement(array); int lowestIndex = example.findIndexOfElement(array, lowestElement); System.out.println( "Lowest element: " + lowestElement); System.out.println( "Index of the Lowest element: " + lowestIndex); } } |
You can compile and execute the above code with the following commands:
1 2 | javac LowestElementTwoStepExample.java java LowestElementTwoStepExample |
The output for the above commands when executed is shown below:
1 2 3 4 5 | bhagvanarch@Bhagvans-MacBook-Air index_lowest_18_Aug % javac LowestElementTwoStepExample.java bhagvanarch@Bhagvans-MacBook-Air index_lowest_18_Aug % java LowestElementTwoStepExample Lowest element: 1 Index of the Lowest element: 1 bhagvanarch@Bhagvans-MacBook-Air index_lowest_18_Aug % |
PseudoCode for Two-step Algorithm to find in Java array, the minimum element position:
Step 1: Finding the Smallest Element:
- The
findSmallestElement()
method iterates through the array to find the smallest element. - It initializes
minElement
to the first element of the array and compares each subsequent element withminElement
. If a smaller element is found, it updatesminElement
. - Finally, the method returns the smallest element.
Step 2: Finding the Index of the Smallest Element:
- The
findIndexOfElement()
method takes the array and the smallest element found in the first step as input. - It iterates through the array to find the first occurrence of the smallest element and returns its index.
Main Method:
- The
main()
method demonstrates how to use the two-step approach. - It first finds the smallest element in the array and then finds its index.
- Finally, it prints the smallest element and its index.
Example Output for the program to find the minimum element position in Java array:
For the array {7, 1, 3, 1, 5, 9, 8, 6},
, this code will print:
1 2 | Smallest element: 1 Index of the smallest element: 1 |
First Occurrence: The findIndexOfElement()
method returns the index of the first occurrence of the smallest element. If the smallest element appears multiple times, only the first index is returned.
Now let us look at the efficiency of this approach. This approach is less efficient compared to a single-step method because it traverses the array twice—once to find the smallest element and once to find its index. The time complexity is O(2n)
(which simplifies to O(n)
).
4. Primitive Streams
In Java, primitive streams are a specialized version of streams that are designed to work with primitive data types (int
, long
, and double
). These streams help avoid the overhead of boxing and unboxing that occurs when using the regular Stream<T>
with wrapper classes (e.g., Integer
, Long
, Double
).
Types of Primitive Streams:
- IntStream: For
int
values. - LongStream: For
long
values. - DoubleStream: For
double
values.
Key Features of Primitive Streams:
- Specialized operations: Methods like
sum()
,average()
,min()
,max()
, etc., are available to perform operations directly on primitive values. - Avoids boxing: Since primitive streams work with primitives directly, there’s no need to convert between primitives and their wrapper types (e.g.,
int
vs.Integer
), which improves performance. - Support for range generation: Primitive streams provide methods like
range()
andrangeClosed()
for generating ranges of numbers.
Example Usage:
1. Creating a Primitive Stream
- From a Collection:
1 2 | List numbers = Arrays.asList( 1 , 2 , 3 , 4 , 5 ); IntStream intStream = numbers.stream().mapToInt(Integer::intValue); |
- From a Range:
1 2 | IntStream rangeStream = IntStream.range( 1 , 5 ); LongStream longRangeStream = LongStream.rangeClosed( 1 , 5 ); |
- From an Array:
1 2 | int [] arr = { 1 , 2 , 3 , 4 , 5 }; IntStream arrayStream = Arrays.stream(arr); |
2. Operations on Primitive Streams
- Aggregation:
1 2 3 | int sum = IntStream.of( 1 , 2 , 3 , 4 , 5 ).sum(); double average = IntStream.of( 1 , 2 , 3 , 4 , 5 ).average().orElse( 0.0 ); int min = IntStream.of( 1 , 2 , 3 , 4 , 5 ).min().orElse(Integer.MAX_VALUE); |
- Reduction:
1 | int product = IntStream.of( 1 , 2 , 3 , 4 ).reduce( 1 , (a, b) -> a * b); |
- Mapping:
1 | IntStream squares = IntStream.range( 1 , 5 ).map(n -> n * n); |
3. Collecting Results:
You can collect primitive streams into collections or arrays:
- To a List:
1 | List list = IntStream.range( 1 , 5 ).boxed().collect(Collectors.toList()); |
- To an Array:
1 | int [] arr = IntStream.range( 1 , 5 ).toArray(); |
Conversions Between Streams
- Converting from a primitive stream to a boxed stream:
1 | Stream boxedStream = IntStream.range( 1 , 5 ).boxed(); |
- Converting from a boxed stream to a primitive stream:
1 | IntStream intStream = Stream.of( 1 , 2 , 3 , 4 ).mapToInt(Integer::intValue) |
Primitive streams in Java (IntStream
, LongStream
, DoubleStream
) provide optimized ways to work with primitive data types by avoiding boxing overhead. They offer specialized operations for aggregation, mapping, and reductions, making them suitable for numeric computations and operations directly on primitives.
Finding the minimum value position in an Array using Streams
Let us look at using Streams to find the minimum value and index in the array. The code is shown below:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 | import java.util.stream.IntStream; import java.util.Comparator; import java.util.Optional; public class LowestElementStreams { public static void main(String[] args) { int [] test_array = { 7 , 1 , 3 , 12 , 5 , 9 , 8 , 6 }; int minValue= IntStream.of(test_array).min().getAsInt(); System.out.println( " Value of the lowest value is :" + minValue); } } |
You can compile and execute the above code with the following commands:
1 2 | javac LowestElementStreams.java java LowestElementStreams |
The output for the above commands when executed is shown below:
1 2 3 | bhagvanarch@Bhagvans-MacBook-Air index_lowest_18_Aug % javac LowestElementStreams.java bhagvanarch@Bhagvans-MacBook-Air index_lowest_18_Aug % java LowestElementStreams Value of the lowest value is :1 |
5. Arrays and Reference Types
In Java, arrays are objects that store elements of a specified type, which can be either primitive types (e.g., int
, double
) or reference types (e.g., String
, custom objects). Understanding how arrays work with reference types is crucial for grasping memory management and object manipulation in Java.
Key Concepts of Java Arrays with Reference Types:
- Arrays Are Objects:
- In Java, arrays themselves are reference types, regardless of whether they hold primitives or objects.
- When you declare an array, memory is allocated for the array object itself, including space for reference to the elements (if it’s an array of reference types).
1 | String[] names = new String[ 5 ]; |
- Elements Are References:
- When dealing with arrays of reference types, each element of the array holds a reference (or pointer) to an object, not the actual object itself.
- If you modify the object that an element of the array points to, all references to that object will see the change.
1 2 3 4 5 6 7 8 9 | String[] names = new String[ 3 ]; names[ 0 ] = new String( "Alice" ); names[ 1 ] = new String( "Bob" ); names[ 2 ] = names[ 0 ]; System.out.println(names[ 2 ]); names[ 0 ] = "Charlie" ; System.out.println(names[ 2 ]); |
- Array Initialization:
- When an array of reference types is created, its elements are initially set to
null
by default, because no objects are assigned yet.
1 2 | String[] names = new String[ 5 ]; System.out.println(names[ 0 ]); |
- Shallow Copy vs. Deep Copy:
- A shallow copy of an array copies the references, not the objects themselves. Therefore, changes to the objects in the copied array will be reflected in the original array, as they share the same references.
- A deep copy involves creating new instances of the objects and copying their values, so the original and copied arrays do not affect each other.
01 02 03 04 05 06 07 08 09 10 | String[] original = { "Alice" , "Bob" }; String[] copy = original; copy[ 0 ] = "Charlie" ; System.out.println(original[ 0 ]); String[] deepCopy = Arrays.copyOf(original, original.length); deepCopy[ 0 ] = "David" ; System.out.println(original[ 0 ]); |
- Null References:
- Since elements of an array of reference types can be
null
, you need to be careful when accessing or manipulating array elements to avoidNullPointerException
.
1 2 | String[] names = new String[ 5 ]; names[ 0 ].length(); |
- An array of Objects:
- You can create arrays of custom objects. The principles remain the same: the array holds references to these objects.
01 02 03 04 05 06 07 08 09 10 11 12 | class Person { String name; Person(String name) { this .name = name; } } Person[] people = new Person[ 2 ]; people[ 0 ] = new Person( "Alice" ); people[ 1 ] = new Person( "Bob" ); System.out.println(people[ 0 ].name); |
Key Points:
- Arrays are reference types: Even when storing primitive types, arrays are objects in Java.
- Reference semantics: When working with arrays of objects, each element holds a reference to an object, not the object itself. This means changes to objects via the array will affect the original object if referenced elsewhere.
- Initialization: Arrays of reference types are initialized with
null
values by default. - Copying: Shallow copying an array will copy the references, while deep copying requires duplicating the objects.
Understanding how arrays and reference types interact is fundamental in Java, especially for working with complex data structures and ensuring proper memory management.
Finding the minimum value position in an Array using Arrays
Let us look at using Arrays to find the minimum value and index in the array. The code is shown below:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | import java.util.Arrays; import java.util.Collections; import java.util.List; import java.util.ArrayList; public class LowestArraysValue { public static void main(String[] args) { int [] arr = { 7 , 1 , 3 , 12 , 5 , 9 , 8 , 6 }; int minValue = Arrays.stream(arr).min().getAsInt(); System.out.println( "Minimum Value is : " + minValue); List intList = new ArrayList(arr.length); for ( int i : arr) { intList.add(i); } System.out.println( "Index of the lowest value element: " + intList.indexOf(minValue)); } } |
You can compile and execute the above code with the following commands:
1 2 | javac LowestArraysValue.java java LowestArraysValue |
The output for the above commands when executed is shown below:
1 2 3 4 5 | bhagvanarch@Bhagvans-MacBook-Air index_lowest_18_Aug % javac LowestArraysValue.java bhagvanarch@Bhagvans-MacBook-Air index_lowest_18_Aug % java LowestArraysValue Minimum Value is : 1 Index of the lowest value element: 1 bhagvanarch@Bhagvans-MacBook-Air index_lowest_18_Aug % |
6. Indexes in Streams
Java Streams API doesn’t directly provide access to element indexes because streams are designed to operate on sequences of elements abstracted from their underlying storage. However, there are several approaches you can use to work with indexes when using Java streams.
Common Approaches for Handling Indexes in Streams
1. Using IntStream.range()
:
If you need to work with both the element and its index, you can use IntStream.range()
to generate a stream of indexes and then map each index to the corresponding element in the original collection or array.
Example:
1 2 3 4 | List list = Arrays.asList( "apple" , "banana" , "cherry" ); IntStream.range( 0 , list.size()) .forEach(i -> System.out.println( "Index: " + i + ", Element: " + list.get(i))); |
2. Pairing Elements with Indexes Using Stream.map()
:
You can create pairs (like Map.Entry<Integer, T>
) to hold both the index and the element.
Example:
1 2 3 4 5 | List list = Arrays.asList( "apple" , "banana" , "cherry" ); IntStream.range( 0 , list.size()) .mapToObj(i -> Map.entry(i, list.get(i))) .forEach(entry -> System.out.println( "Index: " + entry.getKey() + ", Element: " + entry.getValue())); |
This creates a stream of Map.Entry<Integer, T>
, where Integer
is the index, and T
is the element.
3. Zipping with StreamEx
(from the streamex
library):
If you are using the StreamEx
library (an extension of the Java Streams API), you can zip a stream with an index directly.
Example:
1 2 3 4 5 | List list = Arrays.asList( "apple" , "banana" , "cherry" ); StreamEx.of(list) .zipWith(IntStream.range( 0 , list.size()).boxed(), (value, index) -> Map.entry(index, value)) .forEach(entry -> System.out.println( "Index: " + entry.getKey() + ", Element: " + entry.getValue())); |
This approach is more concise and cleaner if you’re open to using an external library.
4. Using AtomicInteger (or other mutable objects):
You can use a mutable integer, such as AtomicInteger
, to track the index within the stream operations.
Example:
1 2 3 4 5 6 | List list = Arrays.asList( "apple" , "banana" , "cherry" ); AtomicInteger index = new AtomicInteger( 0 ); list.stream() .forEach(element -> System.out.println( "Index: " + index.getAndIncrement() + ", Element: " + element)); |
While this approach works, it’s generally not considered the most idiomatic way to work with streams since it involves mutable state, which is typically avoided in functional programming styles.
Key Points:
- Streams abstract away indexes, focusing on element processing rather than element location.
- Use
IntStream.range()
to generate a stream of indexes and pair them with elements. - Libraries like
StreamEx
provide more concise ways to zip streams with indexes. - Mutable objects like
AtomicInteger
can also be used, but they are less idiomatic and should be used cautiously.
Using these techniques, you can work with element indexes in Java streams while adhering to the functional style encouraged by the Streams API.
Finding the minimum value position in an Array using indexes of Streams
Let us look at using indexes of Streams to find the minimum value and index in the array. The code is shown below:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 | import java.util.stream.IntStream; import java.util.Comparator; import java.util.Optional; public class LowestElementStreams { public static void main(String[] args) { int [] test_array = { 7 , 1 , 3 , 12 , 5 , 9 , 8 , 6 }; int minValue= IntStream.of(test_array).min().getAsInt(); System.out.println( " Value of the lowest value is :" + minValue); Optional minIndexArr = IntStream.range( 0 , test_array.length).boxed() .min(Comparator.comparingInt(i -> test_array[i])); System.out.println( "Index of the lowest value element: " + minIndexArr.get()); } } |
You can compile and execute the above code with the following commands:
1 2 | javac LowestElementStreams.java java LowestElementStreams |
The output for the above commands when executed is shown below:
1 2 3 4 | bhagvanarch@Bhagvans-MacBook-Air index_lowest_18_Aug % javac LowestElementStreams.java bhagvanarch@Bhagvans-MacBook-Air index_lowest_18_Aug % java LowestElementStreams Value of the lowest value is :1 Index of the lowest value element: 1 |
7. Conclusion
In this tutorial, we’ve explored how to find the index of the smallest element in an array, a fundamental task in programming. Whether working with small datasets or handling large arrays in complex algorithms, this operation is crucial for tasks such as sorting, searching, and optimization. It is common in Java to encounter a method which finds in Java array, the minimum element position.
By iterating through the array and comparing each element to the current minimum, we can efficiently determine the index of the smallest value. This technique is simple yet powerful, forming the basis for more advanced algorithms. The two-step process helps in finding the minimum first and then the index of the smallest element in an array. We looked at Java Arrays, Streams, and indexes in Streams. Examples were presented demonstrating the usage of them to find the minimum element position in an array.
With this knowledge, you can now confidently implement this logic in your programs, helping you solve problems that involve locating and processing minimum values. Happy coding! You can find the code for this tutorial below from the Download section.
8. Download
You can download the full source code of this example here: Java Array Minimum position – Finding the min element