Java Flatten 2D array Into 1D Array
Arrays are the most basic data structures in any language. Although we don’t work on them directly in most cases, knowing how to manipulate them efficiently can dramatically improve our code.In this tutorial, we’ll learn how to convert a two-dimensional array into one-dimensional, commonly known as flattening. For example, we’ll turn { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} } into {1, 2, 3, 4, 5, 6, 7, 8, 9}.Although we’ll be working with two-dimensional arrays, the ideas outlined in this tutorial can be applied to arrays with any dimensions. In this article, we’ll use an array of primitive integers as an example, but the ideas can be applied to any array. We will look In java, flatten a 2D array into 1D array.
1. Overview
In java, flatten a 2D array into a 1D array is a common requirement. The basic idea is to iterate through the elements of the 2D array and store them sequentially in a new 1D array. You can use Loops, Lists, or Streams in java to convert a 2D array into a 1D array.
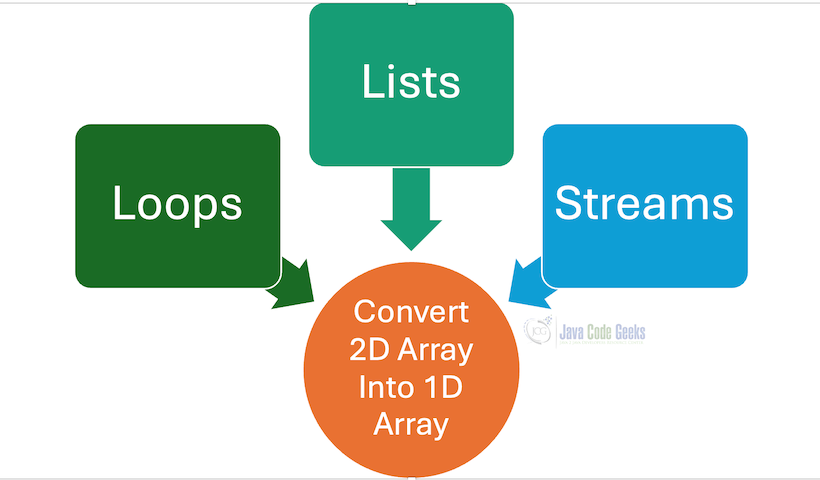
Now let us look at different methods In Java, flatten a 2D array into 1D Array.
2. Loops and Primitive Arrays in java – flatten a 2D array
Here’s how you can convert a 2D array into a 1D array in Java using loops and primitive arrays:
Let’s say you have a 2D array like this:
int[][] exarray2D = { {1, 2, 3}, {4, 5, 6},{7, 8, 9}};
You want to convert it into a 1D array:int[] exarray1D = new int[9];
.The size should be the total number of elements in the 2D array.
Using Nested Loops In Java – flatten a 2D array
1. Calculate the total number of elements:
– This is straightforward if the 2D array is not jagged (i.e., all rows have the same length).
2. Flatten the 2D array:
– Use nested loops to iterate through each element of the 2D array and add them to the 1D array.
Here’s the complete code:
public class FlattenArrayExample { public static void main(String[] args) { int[][] exarray2D = { {1, 2, 3}, {4, 5, 6},{7, 8, 9}}; int totalElements = 0; for (int[] row : exarray2D) { totalElements += row.length; } int[] exarray1D = new int[totalElements]; int index = 0; for (int[] row : exarray2D) { for (int element : row) { exarray1D[index++] = element; } } for (int element : exarray1D) { System.out.print(element + " "); } } }
You can compile and execute the above code with the following commands:
javac FlattenArrayExample.java java FlattenArrayExample
The output for the above commands when executed is shown below:
bhagvanarch@Bhagvans-MacBook-Air convert_2d_to_1d % javac FlattenArrayExample.java bhagvanarch@Bhagvans-MacBook-Air convert_2d_to_1d % java FlattenArrayExample 1 2 3 4 5 6 7 8 9
Explanation
1. Calculate Total Elements:
– The first loop calculates the total number of elements in the 2D array.
2. Flattening Process:
– The nested loops iterate through each element of the 2D array.
– Each element is added to the 1D array, and the index is incremented accordingly.
This method ensures that all elements from the 2D array are transferred to the 1D array in the correct order.
3. Lists in java – flatten a 2D array
Using lists in Java can make the process of converting a 2D array into a 1D array more flexible and convenient. Here’s how you can do it:
Let’s start with a 2D array:
int[][] exarray2D = { {1, 2, 3}, {4, 5, 6},{7, 8, 9}};
Using Lists
1. Create a List to Hold the Elements:
– Use an ArrayList
to store the elements temporarily.
2. Flatten the 2D Array:
– Iterate through each element of the 2D array and add them to the list.
3. Convert the List to a 1D Array:
– Use the toArray
method to convert the list back to a 1D array.
Here’s the complete code:
import java.util.ArrayList; import java.util.List; public class FlattenArrayListExample { public static void main(String[] args) { int[][] exarray2D = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} }; List exlist = new ArrayList(); for (int[] row : exarray2D) { for (int element : row) { exlist.add(element); } } int[] exarray1D = exlist.stream().mapToInt(Integer::intValue).toArray(); for (int element : exarray1D) { System.out.print(element + " "); } } }
You can compile and execute the above code with the following commands:
javac FlattenArrayListExample.java java FlattenArrayListExample
The output for the above commands when executed is shown below:
bhagvanarch@Bhagvans-MacBook-Air convert_2d_to_1d % javac FlattenArrayListExample.java bhagvanarch@Bhagvans-MacBook-Air convert_2d_to_1d % java FlattenArrayListExample 1 2 3 4 5 6 7 8 9
Explanation
1. Create a List:
– An ArrayList
is used to store the elements of the 2D array temporarily.
2. Flattening Process:
– Nested loops iterate through each element of the 2D array and add them to the list.
3. Convert List to Array:
– The stream
method is used to convert the list to an array of integers.
This method is particularly useful when you need the flexibility of a list during the flattening process and then want to convert it back to a primitive array for performance reasons.
4. Stream API
Using the Streams API in Java is a modern and efficient way to convert a 2D array into a 1D array. Here’s how you can do it:
Let’s start with a 2D array:
int[][] exarray2D = { {1, 2, 3}, {4, 5, 6},{7, 8, 9}};
Using Streams API
1. Convert the 2D Array to a Stream of Rows:
– Use Arrays.stream()
to create a stream of the rows of the 2D array.
2. Flatten the Stream:
– Use flatMapToInt()
to flatten the stream of rows into a single stream of integers.
3. Convert the Stream to a 1D Array:
– Use the toArray()
method to convert the flattened stream back to a 1D array.
Here’s the complete code:
import java.util.Arrays; import java.util.stream.IntStream; public class FlattenArrayStreamsExample { public static void main(String[] args) { int[][] exarray2D = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} }; int[] exarray1D = Arrays.stream(exarray2D) .flatMapToInt(Arrays::stream) .toArray(); System.out.println(Arrays.toString(exarray1D)); } }
You can compile and execute the above code with the following commands:
javac FlattenArrayStreamsExample.java java FlattenArrayStreamsExample
The output for the above commands when executed is shown below:
bhagvanarch@Bhagvans-MacBook-Air convert_2d_to_1d % javac FlattenArrayStreamsExample.java bhagvanarch@Bhagvans-MacBook-Air convert_2d_to_1d % java FlattenArrayStreamsExample [1, 2, 3, 4, 5, 6, 7, 8, 9]
Explanation
1. Convert to Stream of Rows:
– Arrays.stream(array2D)
converts the 2D array into a stream where each element is a row (an array of integers).
2. Flatten the Stream:
– flatMapToInt(Arrays::stream)
takes each row (which is an array) and converts it into an IntStream
. The flatMapToInt
method then flattens these streams into a single IntStream
.
3. Convert to 1D Array:
– toArray()
converts the flattened IntStream
into a 1D array of integers.
This method is concise and leverages the power of the Streams API to handle the conversion efficiently.
5. Conclusion
Converting a 2D array into a 1D array in Java is a fundamental task that can be approached in various ways, each with its advantages. Here’s a summary of the methods we’ve covered:
1. Using Nested Loops: This method is straightforward and involves iterating through each element of the 2D array and adding them to a 1D array.
2. Using Lists: Lists provide flexibility and ease of use. You can add elements dynamically and convert the list to an array when needed.
3. Using Streams API: The Streams API offers a modern and concise way to flatten a 2D array. It leverages functional programming concepts to achieve the task efficiently.
Key Takeaways
- Nested Loops: Best for simple and clear implementations.
- Lists: Useful when you need dynamic resizing and flexibility.
- Streams API: Ideal for concise and functional programming approaches.
Each method has its use case, and the choice depends on the specific requirements of your application. Understanding these different approaches will help you choose the most appropriate one for your needs.
6. Download
You can download the full source code of this example here: Remove All Characters Before a Specific Character in Java