Hilla Intro – Tutorial
Hilla is a cutting-edge web framework that enables developers to build full-stack applications seamlessly by combining Java backend services with a modern React frontend. It is designed to provide a smooth development experience, leveraging the strengths of both Java and TypeScript to create robust, scalable, and efficient web applications. This tutorial is about Hilla Intro.
With Hilla, developers can harness the power of Spring Boot for backend development while simultaneously utilizing the dynamic capabilities of React for the front end. This integration allows for real-time, type-safe communication between the front end and back end, simplifying the development process and reducing the likelihood of errors.
One of the standout features of Hilla is its automatic generation of TypeScript services and data types from Java code. This end-to-end type safety ensures that developers can work confidently, knowing that the data structures and methods they use are consistent across both the client and server sides. Additionally, Hilla supports shared validation logic, which helps maintain data integrity and consistency throughout the application.
Hilla also includes a rich set of UI components tailored for complex, data-driven business applications. These components, combined with the flexibility of React, allow developers to build intuitive and interactive user interfaces quickly.
In essence, Hilla provides a comprehensive solution for modern web development, offering a streamlined approach to building full-stack applications with the reliability of Java and the responsiveness of React. Whether you are a seasoned developer or just starting, Hilla empowers you to create high-performance, feature-rich web applications easily.
1. Overview
Welcome to the Hilla Framework tutorial! Hilla is a modern full-stack web framework that seamlessly integrates a React TypeScript frontend with a Spring Boot backend, providing a powerful solution for Java developers to create dynamic and interactive web applications.
In this tutorial, we will cover the following key topics:
- Getting Started with Hilla: An introduction to the core features and advantages of using the Hilla Framework.
- Setting Up the Development Environment: Detailed instructions on installing and configuring Hilla, including setting up Spring Boot and React.
- Creating Your First Hilla Project: Step-by-step guide to creating a new Hilla project using Spring Initializr or integrating Hilla into an existing Spring Boot application.
- Building User Interfaces with React: Techniques for developing responsive and interactive user interfaces using React components and TypeScript.
- Integrating Backend Services: How to connect your React frontend with backend Java services, ensuring smooth data flow and type-safe communication.
- Utilizing Vaadin Components: Leveraging the Vaadin component library to enhance your application’s UI with ready-to-use, high-quality components.
- Deploying and Maintaining Your Application: Best practices for deploying your Hilla application and tips for ongoing maintenance and updates.
By the end of this tutorial, you will have a comprehensive understanding of the Hilla Framework and the skills to build sophisticated full-stack web applications that combine the flexibility of React with the robustness of Java. Let’s dive in and start building with Hilla!
2. Creating a Hilla Project
Creating a Hilla project is an exciting journey into the world of full-stack development that combines the power of Java with the flexibility of React. To get started, you’ll first set up your development environment by installing Java Development Kit (JDK), Node.js, and your preferred IDE, such as IntelliJ IDEA or Visual Studio Code.
Next, create a new Spring Boot project using Spring Initializr or modify an existing one to include Hilla’s necessary dependencies. You’ll then configure your project to support React by setting up the frontend structure within your Spring Boot application.
With the environment ready, you can build your user interface by creating React components in TypeScript. You’ll define views and connect them to backend services using Hilla’s type-safe RPC mechanisms, ensuring smooth data flow and interaction between the front end and backend.
Creating a Hilla project involves leveraging Vaadin’s extensive UI component library to enhance your application’s interface with pre-built, high-quality components. As you develop, you’ll implement form validation and security measures to maintain data integrity and protect your application.
Finally, deploying your Hilla project involves packaging your application and setting it up on a server or cloud platform, followed by continuous monitoring and updates to ensure optimal performance and user satisfaction.
Creating a Hilla project not only enhances your skills in modern web development but also enables you to build robust, scalable, and interactive web applications efficiently.
2.1 Hilla Project
Let us look at the application properties for the implementation:
vaadin.launch-browser=true spring.application.name=example
Let us now look at the project structure. The screenshot below shows the example Hilla Project.
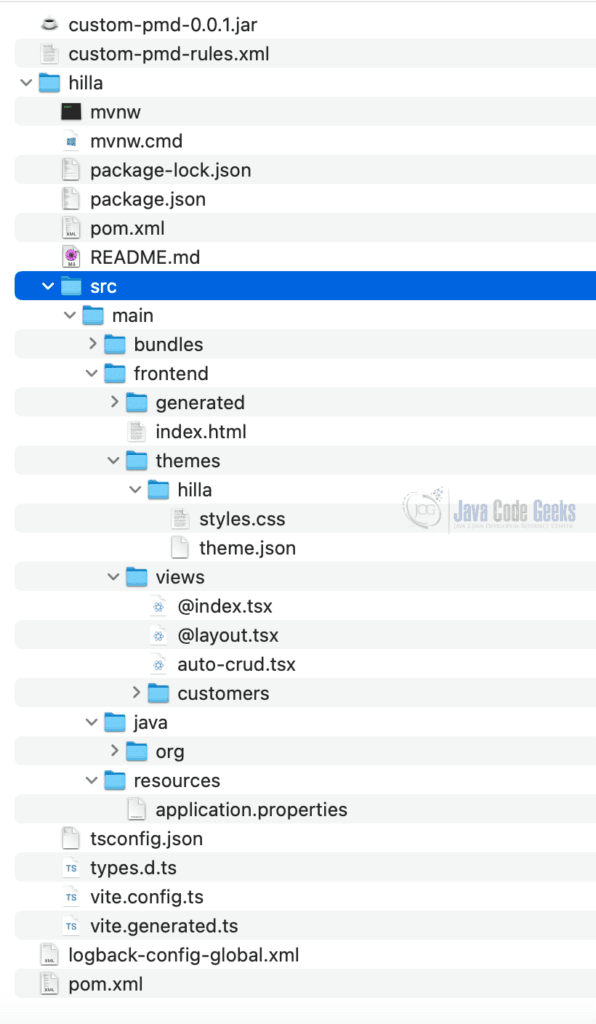
Hilla – Project Structure
When developing a Hilla framework-based application, it’s essential to maintain a clear and organized project structure to ensure smooth development and easy maintenance.
2.1.1 Backend
src/main/java/com/example/myapp/
: This is the main package for your Java backend code. Within this package, you can organize your classes into sub-packages:service/
: Contains the service layer classes, where the business logic is implemented.controller/
: Contains REST controllers that handle HTTP requests and responses.repository/
: Contains repository interfaces for data access, typically extending Spring Data JPA repositories.entity/
: Contains entity classes representing database tables.dto/
: Contains Data Transfer Objects used for transferring data between the backend and frontend.MyApplication.java
: The main entry point of the Spring Boot application.
src/main/resources/
: Contains configuration files and static resources for the backend.application.properties
: The main configuration file for the Spring Boot application.
2.1.2 Frontend
src/
: The main source directory for your React frontend code.components/
: Contains reusable React components.views/
: Contains view components that correspond to different routes in your application.App.tsx
: The main application component.index.tsx
: The entry point of the React application.services/
: Contains TypeScript services for making API calls to the backend.
public/
: Contains public assets for the frontend.index.html
: The main HTML file for your React application.
package.json
: Contains the list of npm dependencies and scripts for the frontend.tsconfig.json
: The TypeScript configuration file.webpack.config.js
: The Webpack configuration file for bundling the frontend assets.
2.1.3 Root
pom.xml
: The Maven configuration file for the entire project.README.md
: A file containing information about the project.
This structure ensures a clean separation between backend and frontend code, making it easier to manage and scale your application.
2.2 Hilla Project Dependencies
To set up a Hilla framework-based application using Spring Boot, you’ll need to include specific dependencies in your pom.xml
file. Here are the essential dependencies:
2.2.1 Backend Dependencies
- Spring Boot Starter: This is the core dependency for any Spring Boot application.xml
- Spring Boot Starter Web: Required for building web applications, including RESTful services.xml
- Spring Boot Starter Data JPA: For working with JPA and databases.xml
- Hilla: The main dependency for Hilla framework.xml
- Database Driver: Depending on your choice of database (e.g., H2, MySQL, PostgreSQL).
- For H2
- For MySQL
2.2.2 Frontend Dependencies
For the frontend of a Hilla application, you need to manage dependencies using npm
in the package.json
file.
- React: Core React library
- Hilla Client: Hilla-specific client-side library
- Other Required Libraries: Depending on your application needs, you might include libraries for state management (e.g., Redux), routing (e.g., React Router), and UI components.
2.2.3 Build Plugins
To build and package your application, you’ll need specific plugins in your pom.xml
:
- Spring Boot Maven Plugin
- Frontend Maven Plugin
With these dependencies and plugins set up, you’ll be ready to develop and build your Hilla framework-based application using Spring Boot
3. Starting the Application
Starting your Hilla application is an essential step that brings together the backend and frontend components of your full-stack project. To begin, navigate to the root directory of your Hilla project in your terminal or command prompt. Use the command `mvn spring-boot:run` to start the Spring Boot backend, which will initialize the application server and set up the backend environment.
Simultaneously, open a new terminal window and navigate to the frontend directory within your project. Use the command `npm start` to launch the React development server, which compiles the frontend code and starts a live-reloading server for your React components.
Once both servers are running, open your web browser and navigate to `http://localhost:8080` to access your Hilla application. Here, you’ll see your React-based user interface interacting seamlessly with the Spring Boot backend. You can now start testing and refining your application, ensuring that all components work together as intended.
As you develop, make sure to monitor both the backend and frontend servers for any errors or issues, and utilize the live-reloading feature to see changes in real time. This approach ensures a smooth development experience and allows you to build a robust, full-stack web application with Hilla.
Hilla Project Execution
You can compile and execute the above code with the following commands:
export JAVA_HOME=/Library/Java/JavaVirtualMachines/openjdk.jdk/Contents/Home mvn clean ./mvnw package -Pproduction
The output for the above commands when executed is shown below:
bhagvanarch@Bhagvans-MacBook-Air hilla % ./mvnw package -Pproduction [INFO] Scanning for projects... [INFO] gitflow-incremental-builder is disabled. [INFO] [INFO] --------------------------------------------------- [INFO] Building hilla 0.0.1-SNAPSHOT [INFO] from pom.xml [INFO] --------------------------------[ jar ]--------------------------------- [WARNING] Parameter 'sourceEncoding' is unknown for plugin 'maven-pmd-plugin:3.23.0:check (default)' [WARNING] Parameter 'sourceEncoding' is unknown for plugin 'maven-pmd-plugin:3.23.0:pmd (pmd)' [INFO] [INFO] --- directory:1.0:directory-of (directories) @ hilla --- [INFO] Directory of org.javacodgeeks:parent-modules set to: /Users/bhagvanarch/kazdesk/javacodegeeks/code/hilla_dec_18_2024 [INFO] [INFO] --- install:3.1.2:install-file (install-jar-lib) @ hilla --- [INFO] Installing /Users/bhagvanarch/kazdesk/javacodegeeks/code/hilla_dec_18_2024/custom-pmd-0.0.1.jar to /Users/bhagvanarch/.m2/repository/org/custom/pmd/custom-pmd/0.0.1/custom-pmd-0.0.1.jar [INFO] Installing /var/folders/jm/7m3hf4f1639_vpyg29093b2h0000gn/T/mvninstall2047103854312298556.pom to /Users/bhagvanarch/.m2/repository/org/custom/pmd/custom-pmd/0.0.1/custom-pmd-0.0.1.pom [INFO] [INFO] --- resources:3.3.0:resources (default-resources) @ hilla --- [INFO] Copying 1 resource [INFO] [INFO] --- compiler:3.12.1:compile (default-compile) @ hilla --- [INFO] Nothing to compile - all classes are up to date. [INFO] [INFO] >>> pmd:3.23.0:check (default) > :pmd @ hilla >>> [INFO] [INFO] --- pmd:3.23.0:pmd (pmd) @ hilla --- [WARNING] Parameter 'aggregate' (user property 'aggregate') is deprecated: since 3.15.0 Use the goals pmd:aggregate-pmd and pmd:aggregate-cpd instead. [INFO] PMD version: 7.0.0 [INFO] Rendering content with org.apache.maven.skins:maven-default-skin:jar:1.3 skin. [INFO] [INFO] <<< pmd:3.23.0:check (default) < :pmd @ hilla <<>> vaadin:24.4.10:prepare-frontend (frontend) > :configure @ hilla >>> [INFO] [INFO] --- vaadin:24.4.10:configure (configure) @ hilla --- [INFO] Reflections took 1007 ms to scan 169 urls, producing 9309 keys and 49253 values [INFO] [INFO] <<< vaadin:24.4.10:prepare-frontend (frontend) < :configure @ hilla <<>> vaadin:24.4.10:build-frontend (frontend) > :configure @ hilla >>> [INFO] [INFO] --- vaadin:24.4.10:configure (configure) @ hilla --- [INFO] Reflections took 303 ms to scan 169 urls, producing 9309 keys and 49253 values [INFO] [INFO] <<< vaadin:24.4.10:build-frontend (frontend) < :configure @ hilla <<< [INFO] [INFO] [INFO] --- vaadin:24.4.10:build-frontend (frontend) @ hilla --- [INFO] Reflections took 311 ms to scan 169 urls, producing 9309 keys and 49253 values [INFO] Reflections took 285 ms to scan 169 urls, producing 9309 keys and 49253 values [INFO] Scanning classes to find frontend configurations and dependencies... [INFO] Visited 5990 classes. Took 1674 ms. [INFO] Checking if a production mode bundle build is needed [INFO] Frontend build requested. [INFO] Skipping `npm install` because the frontend packages are already installed in the folder '/Users/bhagvanarch/kazdesk/javacodegeeks/code/hilla_dec_18_2024/hilla/node_modules' and the hash in the file '/Users/bhagvanarch/kazdesk/javacodegeeks/code/hilla_dec_18_2024/hilla/node_modules/.vaadin/vaadin.json' is the same as in 'package.json' WARN (tsgen) : Component has no properties: com.vaadin.hilla.crud.filter.Filter [INFO] Copying frontend resources from jar files ... [INFO] Visited 168 resources. Took 66 ms. [INFO] Running Vite ... [INFO] Reflections took 504 ms to scan 169 urls, producing 9309 keys and 49253 values [INFO] Reflections took 314 ms to scan 169 urls, producing 9309 keys and 49253 values [INFO] Scanning classes to find frontend configurations and dependencies... [INFO] Visited 5990 classes. Took 1385 ms. [INFO] Build frontend completed in 18854 ms. [INFO] [INFO] --- resources:3.3.0:testResources (default-testResources) @ hilla --- [INFO] skip non existing resourceDirectory /Users/bhagvanarch/kazdesk/javacodegeeks/code/hilla_dec_18_2024/hilla/src/test/resources [INFO] [INFO] --- compiler:3.12.1:testCompile (default-testCompile) @ hilla --- [INFO] No sources to compile [INFO] [INFO] --- surefire:3.2.5:test (default-test) @ hilla --- [INFO] No tests to run. [INFO] [INFO] --- jar:3.4.2:jar (default-jar) @ hilla --- [INFO] Building jar: /Users/bhagvanarch/kazdesk/javacodegeeks/code/hilla_dec_18_2024/hilla/target/hilla-0.0.1-SNAPSHOT.jar [INFO] [INFO] --- spring-boot:3.3.2:repackage (repackage) @ hilla --- [INFO] Replacing main artifact /Users/bhagvanarch/kazdesk/javacodegeeks/code/hilla_dec_18_2024/hilla/target/hilla-0.0.1-SNAPSHOT.jar with repackaged archive, adding nested dependencies in BOOT-INF/. [INFO] The original artifact has been renamed to /Users/bhagvanarch/kazdesk/javacodegeeks/code/hilla_dec_18_2024/hilla/target/hilla-0.0.1-SNAPSHOT.jar.original [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 25.159 s [INFO] Finished at: 2024-12-23T22:21:43+05:30 [INFO] ------------------------------------------------------------------------ bhagvanarch@Bhagvans-MacBook-Air hilla %
4. Calling Server Methods With @BrowserCallable
In the Hilla framework, calling server methods with @BrowserCallable is a powerful feature that facilitates seamless communication between the client side and the server side. @BrowserCallable allows you to define methods in your Java backend that can be invoked directly from the browser using JavaScript.
To get started, annotate the desired server method with `@BrowserCallable`. This tells the Hilla framework that the method can be safely called from the client-side code. Next, expose these methods in your client-side TypeScript code, making them available for use within your React components or any other JavaScript code.
For instance, you might create a method in your backend service to fetch data from a database or perform a calculation. By marking this method with @BrowserCallable, you enable your front end to call this method asynchronously, retrieving the data or result without needing to reload the page or manually handle HTTP requests.
This approach streamlines the development process, allowing you to write cleaner and more maintainable code. It also enhances the user experience by enabling more dynamic and interactive web applications.
Using @BrowserCallable in Hilla is particularly useful for real-time applications where data needs to be frequently updated or where user interactions trigger server-side logic. It bridges the gap between the front end and backend, making it easier to build complex, responsive web applications with a smooth and efficient workflow.
Hilla Data Service: Browser Callable
Let us look at the Java code for the @BrowserCallable implementation:
package org.javacodegeeks.hilla; import com.vaadin.flow.server.auth.AnonymousAllowed; import com.vaadin.hilla.BrowserCallable; import com.vaadin.hilla.crud.CrudRepositoryService; import com.vaadin.hilla.Nonnull; import java.util.List; @BrowserCallable @AnonymousAllowed public class CustomerService extends CrudRepositoryService { @Nonnull public List findAll() { return getRepository().findAll(); } public Customer findById(Long id) { return getRepository().findById(id) .orElseThrow(); } }
The CustomerRepository class is shown below. CustomerRepository is an interface which extends JPARepository.
package org.javacodegeeks.hilla; import org.springframework.data.jpa.repository.JpaRepository; import org.springframework.data.jpa.repository.JpaSpecificationExecutor; public interface CustomerRepository extends JpaRepository, JpaSpecificationExecutor { }
5. Configuring Views and Layouts
Configuring views and layouts in the Hilla framework involves a structured approach to creating an organized and user-friendly interface. Views in Hilla are created using React components written in TypeScript. Each view represents a different page or section of your application, and these views can be structured to display various UI elements and handle user interactions.
To start, you’ll define your main layout, which typically includes common elements like the header, footer, and navigation menu. This layout serves as the container for your views, ensuring consistency across different parts of your application. You can create a main layout component that wraps around your views, allowing for a cohesive design and user experience.
Next, you’ll create individual view components for each page or section of your application. These components will be routed to their respective URLs using React Router, enabling seamless navigation between views. Each view component can include various UI elements and logic to handle user interactions and display data from the backend.
By configuring views and layouts in the Hilla framework, you ensure that your application has a well-organized structure and a consistent look and feel, providing an intuitive and engaging user experience.
Let us now see the Hilla example Layouts.tsx. The layout has the header, and Navigation Link.
import { createMenuItems } from '@vaadin/hilla-file-router/runtime.js'; import { NavLink, Outlet } from 'react-router-dom'; import { Suspense } from 'react'; export default function MainLayout() { return ( <div className="p-m h-full flex flex-col box-border"> <header className="flex gap-m pb-m"> <h1 className="text-l m-0"> CRM App </h1> {createMenuItems().map(({ to, title }) => ( <NavLink to={to} key={to}> {title} </NavLink> ))} </header> <Suspense> <Outlet /> </Suspense> </div> ); }
Now let us see the theme.json. The theme.json has different lumo imports
{ "lumoImports" : [ "typography", "color", "sizing", "spacing", "utility" ] }
Let us see the Landing page in HTML. This is auto-generated by Vaadin.
<!DOCTYPE html> <!-- This file is auto-generated by Vaadin. --> <html> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <style> body, #outlet { height: 100vh; width: 100%; margin: 0; } </style> <!-- index.ts is included here automatically (either by the dev server or during the build) --> </head> <body> <!-- This outlet div is where the views are rendered --> <div id="outlet"></div> </body> </html>
6. Building Forms and Validating Input
Building Forms and Validating Input in Hilla Framework
Creating forms and validating input are fundamental aspects of web development, and the Hilla framework provides robust tools to streamline these tasks. The framework combines the power of Java backend services with the dynamic capabilities of React on the front end, ensuring a seamless experience for developers.
Building Forms
Hilla makes it straightforward to build complex forms with a rich set of UI components. You can utilize Vaadin’s extensive component library, which includes everything from text fields and checkboxes to date pickers and file uploaders. These components are designed to integrate smoothly with React, allowing you to create intuitive and responsive user interfaces.
In a typical Hilla application, you would define your form components in a TypeScript file, binding them to backend services through type-safe APIs generated by Hilla. This ensures that the form elements are dynamically updated based on the backend logic and data models.
Validating Input
Input validation is critical for maintaining data integrity and ensuring a seamless user experience. Hilla supports both client-side and server-side validation, offering a comprehensive approach to handling user input.
Client-Side Validation: You can implement client-side validation directly within your React components using TypeScript. This allows for immediate feedback to users, improving the overall usability of the form. For example, you can check if a text field is empty, verify that an email address is in the correct format, or ensure that a password meets specific criteria.
Server-Side Validation: Hilla enables server-side validation through its integration with Spring Boot. By defining validation logic in your Java backend services, you can ensure that all data submitted through the forms meets your application’s requirements. This is particularly useful for complex validation rules that might involve database checks or other backend logic.
Combining Client-Side and Server-Side Validation
One of the key strengths of Hilla is its ability to combine client-side and server-side validation seamlessly. When a user submits a form, the initial validation can happen on the client side, providing immediate feedback. If the input passes client-side validation, it is then sent to the server, where additional validation checks are performed before processing the data.
Benefits of Using Hilla for Forms and Validation
- Type Safety: The automatic generation of TypeScript services ensures that the form components and validation logic are type-safe, reducing runtime errors.
- Rich UI Components: Vaadin’s component library provides a wide range of pre-built components, making it easier to build sophisticated forms.
- Integrated Validation: By supporting both client-side and server-side validation, Hilla ensures that all user inputs are thoroughly checked, improving data integrity and security.
Overall, the Hilla framework offers a powerful and efficient way to build and validate forms, combining the strengths of Java and TypeScript to deliver a superior development experience.
Let us look at the forms in the Hilla Example -edit.tsx. This form is for creating a customer.
import {Button, TextField} from "@vaadin/react-components"; import {useEffect} from "react"; import {useNavigate, useParams} from "react-router-dom"; import {useForm} from "@vaadin/hilla-react-form"; import {CustomerService} from "Frontend/generated/endpoints"; import CustomerModel from "Frontend/generated/org/javacodegeeks/hilla/CustomerModel"; export default function CustomerEditor() { const {id} = useParams(); const navigate = useNavigate(); const {field, model, submit, read} = useForm(CustomerModel, { onSubmit: async contact => { await CustomerService.save(contact); navigate('/'); } }) async function loadUser(id: number) { read(await CustomerService.findById(id)) } useEffect(() => { if (id) { loadUser(parseInt(id)) } }, [id]); return ( <div className="flex flex-col items-start gap-m"> <TextField label="Name" {...field(model.name)}/> <TextField label="Email" {...field(model.email)}/> <TextField label="Phone" {...field(model.mobile)}/> <div className="flex gap-s"> <Button onClick={submit} theme="primary">Save</Button> <Button onClick={() => navigate('/')} theme="tertiary">Cancel</Button> </div> </div> ); }
The above customer form has the fields – Name, Email, Mobile phone as Textfields. The user fills the form and submits it to the customerService to save a new customer.
7. Automatic CRUD Operations With AutoCrud
Automatic CRUD (Create, Read, Update, Delete) operations with AutoCrud in the Hilla framework simplify the management of data entities within your application. AutoCrud leverages the power of Hilla to automatically generate these essential operations, reducing the amount of boilerplate code developers need to write.
To use AutoCrud, you start by defining your data entities in the backend using Java. These entities represent the structure of the data you want to manage, such as user profiles, orders, or products. Hilla’s AutoCrud then generates the necessary CRUD operations for these entities, including endpoints for creating new records, retrieving existing ones, updating records, and deleting them.
On the frontend, you can interact with these CRUD operations seamlessly using React components and TypeScript. Hilla provides type-safe APIs that allow your React components to call the generated endpoints, ensuring smooth data transactions between the client and server.
AutoCrud streamlines the development process by handling repetitive tasks, enabling you to focus on building the unique features of your application. It also ensures consistency and reliability in data management, making it easier to maintain and scale your application as it grows. With AutoCrud, managing data entities in a Hilla application becomes efficient and straightforward.
Now let us look at autocrud implementation in the example – auto-crud.tsx.
import CustomerModel from 'Frontend/generated/org/javacodegeeks/hilla/CustomerModel'; import { CustomerService } from 'Frontend/generated/endpoints'; import { AutoCrud } from '@vaadin/hilla-react-crud'; export default function AutoCrudView() { return <AutoCrud service={CustomerService} model={CustomerModel} className="h-full" />; }
Autocrud view uses the CustomerModel for rendering the list view with update and delete customer features. Let us look at the Customer Model. The Customer Model is generated from the Customer class in Java.
package org.javacodegeeks.hilla; import jakarta.persistence.Entity; import jakarta.persistence.GeneratedValue; import jakarta.persistence.Id; import jakarta.validation.constraints.Email; import jakarta.validation.constraints.Size; @Entity public class Customer { @Id @GeneratedValue private Long id; @Size(min = 2) private String name; @Email private String email; private String mobile; public Customer() { } public Customer(String name, String email, String mobile) { this.name = name; this.email = email; this.mobile = mobile; } public Long getId() { return id; } public void setId(Long id) { this.id = id; } public @Size(min = 2) String getName() { return name; } public void setName(@Size(min = 2) String name) { this.name = name; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } public String getMobile() { return mobile; } public void setMobile(String mobile) { this.mobile = mobile; } }
Let us now look at the Application Runner for generating data which is CustomerData. CustomerData uses the faker framework to generate 50 customers with name, email, and mobile phone numbers.
package org.javacodegeeks.hilla; import org.springframework.boot.ApplicationArguments; import org.springframework.boot.ApplicationRunner; import org.springframework.stereotype.Component; import com.github.javafaker.Faker; @Component public class CustomerData implements ApplicationRunner { private final CustomerRepository customerRepository; public CustomerData(CustomerRepository customerRepository) { this.customerRepository = customerRepository; } @Override public void run(ApplicationArguments args) { for (int i = 0; i < 50; i++) { Faker faker = new Faker(); String name = faker.name().fullName(); String email = faker.internet().emailAddress(); String mobile = faker.phoneNumber().cellPhone(); customerRepository.save(new Customer(name, email, mobile)); } } }
8. Building for Production
Building for production using the Hilla framework involves several steps to ensure your application is robust, secure, and ready for deployment. Start by optimizing your codebase to remove any unnecessary dependencies and debug statements that might have been used during development. This helps in reducing the application size and improving performance.
The app class, the entry point of the Hilla Application, is shown below. This is a Spring Boot application.
package org.javacodegeeks.hilla; import com.vaadin.flow.component.page.AppShellConfigurator; import com.vaadin.flow.theme.Theme; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication @Theme("hilla") public class CRMApp implements AppShellConfigurator { public static void main(String[] args) { SpringApplication.run(CRMApp.class, args); } }
Next, configure the build process to create production-ready artefacts. For the backend, ensure that your Spring Boot application is correctly configured with necessary profiles and settings for the production environment. For the frontend, use tools like Webpack to bundle and minify your React code, ensuring efficient loading times.
Security is paramount when preparing for production. Implement robust security measures, including HTTPS, to secure data transmission. Validate and sanitize all inputs to prevent injection attacks. Also, configure authentication and authorization mechanisms to protect sensitive data and functionalities.
Monitor your application to detect and address issues quickly. Use logging frameworks to capture and analyze application logs, and set up monitoring tools to track performance and resource usage.
Finally, deploy your application to a reliable server or cloud platform. Ensure that your deployment pipeline is automated for smooth updates and rollbacks. With these steps, your Hilla application will be well-prepared for a production environment, offering users a secure, efficient, and seamless experience.
You can access the application on the browser by clicking on this URL : http://localhost:8080/ The screenshot of the app is show below :
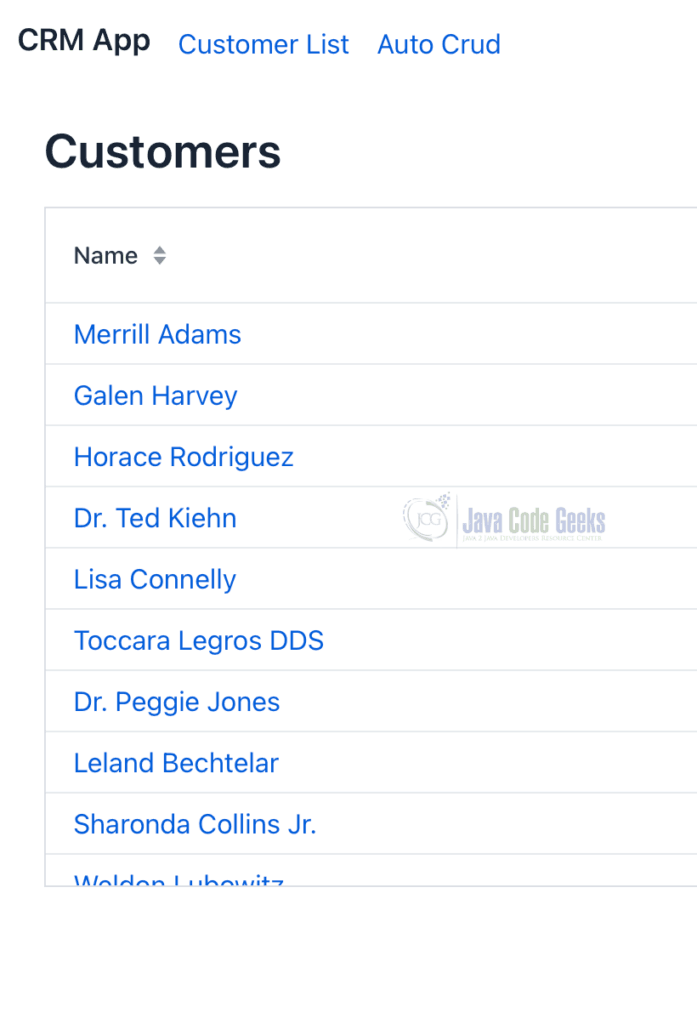
You can edit the customer info by using the edit form. The screenshot of the edit form is shown below:
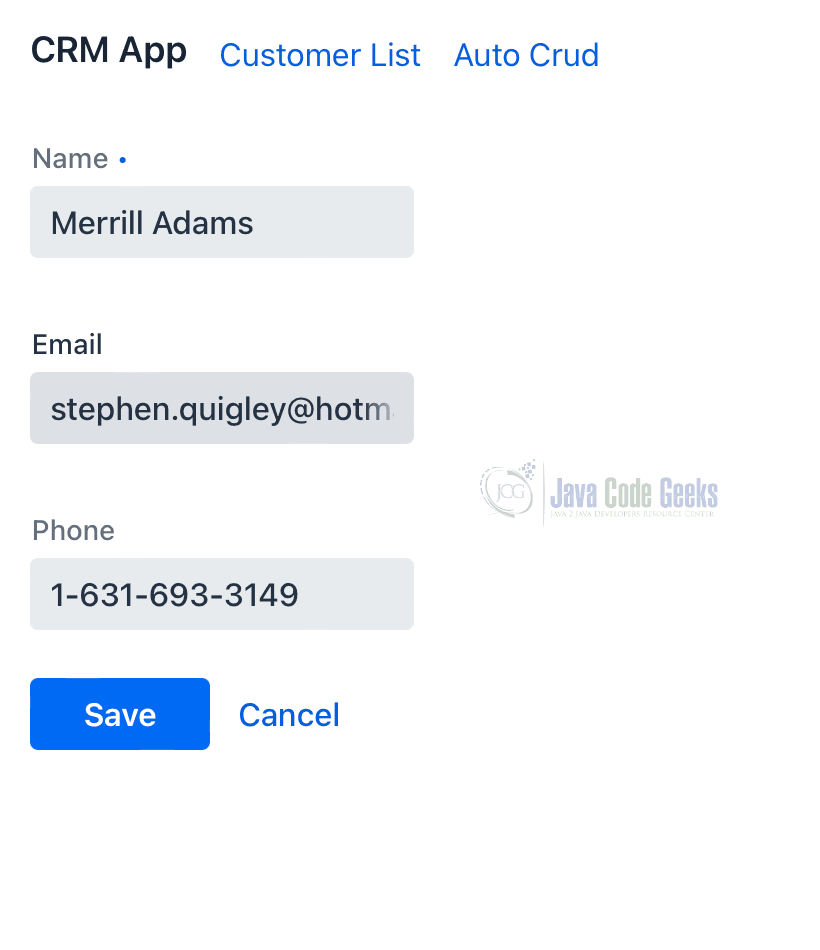
You can create new customer by using the create form. The screenshot of the create customer form is shown below:
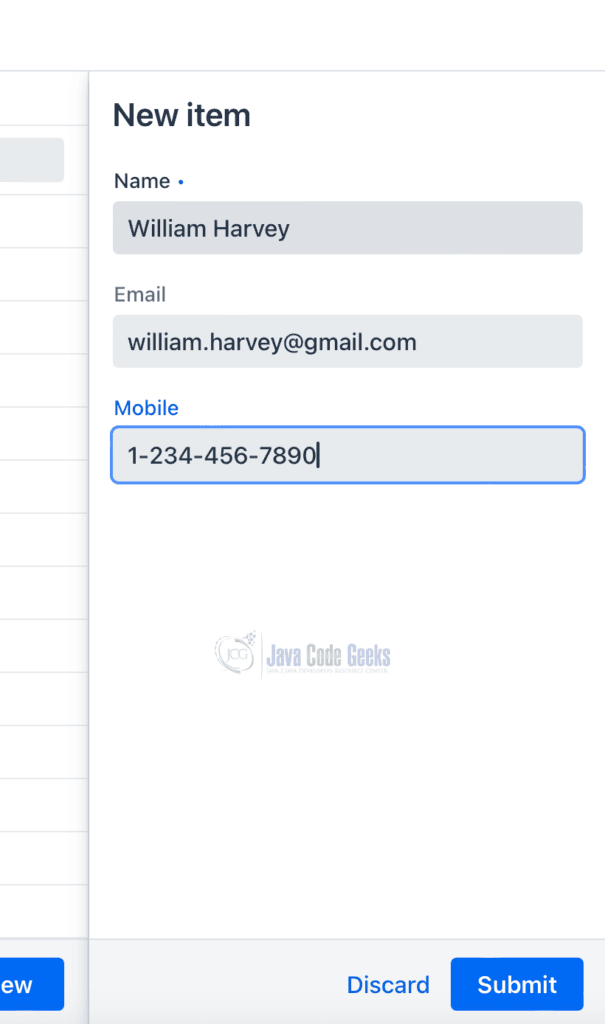
9. Conclusion
In this tutorial, we’ve journeyed through the essentials of the Hilla Framework, exploring its seamless integration of a React TypeScript frontend with a Spring Boot backend. We’ve covered setting up your development environment, creating a new project, building responsive user interfaces, and integrating backend services. Additionally, we’ve delved into advanced topics such as form validation, security, and deploying your production application.
Hilla offers a robust and efficient solution for full-stack web development, combining the strengths of both Java and React. Mastering these concepts allows you to build dynamic, scalable, and secure web applications that meet modern development standards. Keep experimenting and building upon these foundations, and you’ll unlock the full potential of the Hilla Framework in your future projects. Happy coding!
10. Download
You can download the full source code of this example here: Introduction to the Hilla Framework