In today’s software development landscape, ensuring the reliability and accuracy of data interchange formats like JSON is crucial. As applications increasingly rely on JSON for API communication, it becomes imperative to have robust testing mechanisms in place. Enter JsonUnit, a powerful library that simplifies and enhances JSON unit testing. We will be looking at a JsonUnit Assertj-JSON unit test example.
JsonUnit empowers developers to perform comprehensive assertions on JSON data, ensuring that APIs deliver consistent and correct responses. It provides a rich set of tools for comparing JSON structures, validating key-value pairs, and handling complex scenarios. This tutorial will guide you through the essentials of using JsonUnit, from basic assertions to advanced techniques, enabling you to write reliable and maintainable tests for your JSON data.
1. Overview
JsonUnit is a powerful library designed for developers who need to perform unit tests on JSON data. This tutorial covers the essential aspects of using JsonUnit for effective JSON testing. Starting with the basics, you’ll learn how to set up JsonUnit in your project, followed by fundamental assertions for comparing JSON structures and validating key-value pairs.
We’ll delve into advanced features like handling complex JSON scenarios, custom comparison strategies, and integrating JsonUnit with popular testing frameworks such as JUnit. By the end of this tutorial, you’ll have a solid understanding of JsonUnit’s capabilities and be equipped to write reliable, maintainable JSON tests for your applications.
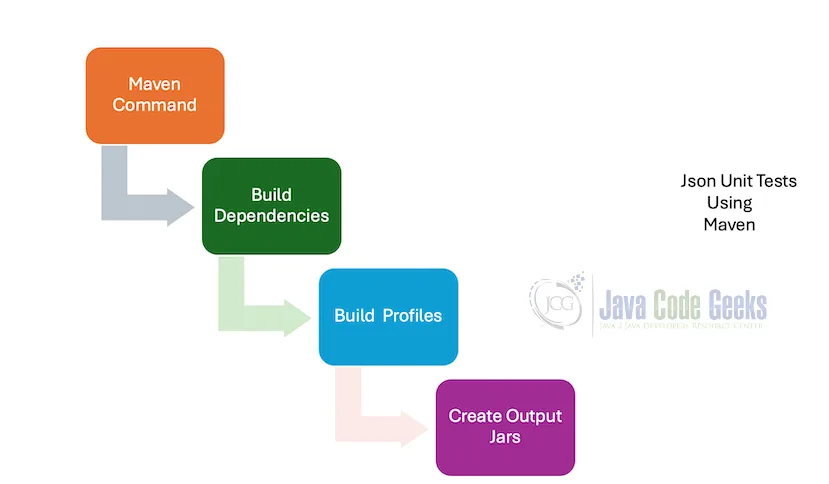
Now let us get started with JSON Unit for JSON Unit test Assertions.
2. Getting Started
Embarking on your journey with JsonUnit is straightforward and rewarding. To get started, follow these steps:
Step 1: Add JsonUnit to Your Project
First, you’ll need to add JsonUnit to your project. If you’re using Maven, include the following dependency in your pom.xml file:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | <? xml version = "1.0" encoding = "UTF-8" ?> < modelVersion >4.0.0</ modelVersion > < artifactId >jsontests</ artifactId > < version >0.1-SNAPSHOT</ version > < name >jsontests</ name > < properties > < json-unit.version >3.5.0</ json-unit.version > < jackson-databind.version >2.18.1</ jackson-databind.version > </ properties > < parent > < groupId >org.javacodegeeks</ groupId > < artifactId >jsonunittest</ artifactId > < version >1.0.0-SNAPSHOT</ version > </ parent > < dependencies > < dependency > < groupId >net.javacrumbs.json-unit</ groupId > < artifactId >json-unit-assertj</ artifactId > < version >${json-unit.version}</ version > < scope >test</ scope > </ dependency > </ project > |
Step 2: Basic Setup
Once you’ve added the dependency, you can start using JsonUnit. Import the necessary dependencies in pom.xml
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 | <? xml version = "1.0" encoding = "UTF-8" ?> < modelVersion >4.0.0</ modelVersion > < artifactId >jsontests</ artifactId > < version >0.1-SNAPSHOT</ version > < name >jsontests</ name > < properties > < json-unit.version >3.5.0</ json-unit.version > < jackson-databind.version >2.18.1</ jackson-databind.version > </ properties > < parent > < groupId >org.javacodegeeks</ groupId > < artifactId >jsonunittest</ artifactId > < version >1.0.0-SNAPSHOT</ version > </ parent > < dependencies > < dependency > < groupId >net.javacrumbs.json-unit</ groupId > < artifactId >json-unit-assertj</ artifactId > < version >${json-unit.version}</ version > < scope >test</ scope > </ dependency > < dependency > < groupId >org.assertj</ groupId > < artifactId >assertj-core</ artifactId > < version >${assertj.version}</ version > < scope >test</ scope > </ dependency > < dependency > < groupId >com.fasterxml.jackson.core</ groupId > < artifactId >jackson-databind</ artifactId > < version >${jackson-databind.version}</ version > < scope >test</ scope > </ dependency > </ dependencies > </ project > |
Step 3: Writing Your First Test
Now, let’s write a basic test to compare JSON string with another JSON Object.
Let us look at the Java code for the implementation:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | package com.baeldung.jsonunit; import static net.javacrumbs.jsonunit.assertj.JsonAssertions.assertThatJson; import static net.javacrumbs.jsonunit.assertj.JsonAssertions.json; import java.util.List; import org.junit.jupiter.api.Test; import net.javacrumbs.jsonunit.core.Option; class JsonUnitAssertionsUnitTest { @Test void whenWeVerifyAJsonObject_thenItContainsKeyValueEntries() { String articleJson = "" " { "name" : "A Guide to Spring Boot" , "tags" : [ "java" , "spring boot" , "backend" ] } "" "; assertThatJson(articleJson).isObject() .containsEntry( "name" , "A Guide to Spring Boot" ) .containsEntry( "tags" , List.of( "java" , "spring boot" , "backend" )); } } |
You can compile and execute the above code with the following commands:
1 2 | mvn package mvn test |
The output for the above commands when executed is shown below:
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 083 084 085 086 087 088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 | bhagvanarch@Bhagvans-MacBook-Air jsontests % mvn package [INFO] Scanning for projects... [INFO] gitflow-incremental-builder is disabled. [INFO] [INFO] ----------------------------------------- [INFO] Building jsontests 0.1-SNAPSHOT [INFO] from pom.xml [INFO] --------------------------------[ jar ]--------------------------------- [WARNING] Parameter 'sourceEncoding' is unknown for plugin 'maven-pmd-plugin:3.26.0:check (default)' [WARNING] Parameter 'sourceEncoding' is unknown for plugin 'maven-pmd-plugin:3.26.0:pmd (pmd)' [INFO] [INFO] --- directory:1.0:directory-of (directories) @ jsontests --- [INFO] Directory of org.javacodegeeks:parent-modules set to: /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest [INFO] [INFO] --- install :3.1.2: install - file ( install -jar-lib) @ jsontests --- [INFO] Installing /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/custom-pmd-0 .0.1.jar to /Users/bhagvanarch/ .m2 /repository/org/baeldung/pmd/custom-pmd/0 .0.1 /custom-pmd-0 .0.1.jar [INFO] Installing /var/folders/jm/7m3hf4f1639_vpyg29093b2h0000gn/T/mvninstall17870915735783281865 .pom to /Users/bhagvanarch/ .m2 /repository/org/baeldung/pmd/custom-pmd/0 .0.1 /custom-pmd-0 .0.1.pom [INFO] [INFO] --- resources:3.3.1:resources (default-resources) @ jsontests --- [INFO] skip non existing resourceDirectory /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/jsonunit/jsontests/src/main/resources [INFO] [INFO] --- compiler:3.13.0:compile (default-compile) @ jsontests --- [INFO] Recompiling the module because of changed source code. [INFO] Compiling 3 source files with javac [debug target 8] to target /classes [WARNING] bootstrap class path not set in conjunction with - source 8 [INFO] [INFO] >>> pmd:3.26.0:check (default) > :pmd @ jsontests >>> [INFO] [INFO] --- pmd:3.26.0:pmd (pmd) @ jsontests --- [WARNING] Parameter 'aggregate' (user property 'aggregate' ) is deprecated: since 3.15.0 Use the goals pmd:aggregate-pmd and pmd:aggregate-cpd instead. See FAQ: Why do I get sometimes false positive and /or false negative violations? for an explanation. [INFO] PMD version: 7.7.0 [INFO] Rendering content with org.apache.maven.skins:maven-fluido-skin:jar:2.0.0-M9 skin [INFO] [INFO] <<< pmd:3.26.0:check (default) < :pmd @ jsontests <<< [INFO] [INFO] [INFO] --- pmd:3.26.0:check (default) @ jsontests --- [WARNING] Parameter 'aggregate' (user property 'aggregate' ) is deprecated: since 3.15.0 Use the goal pmd:aggregate-check or pmd:aggregate-cpd-check instead. [INFO] [INFO] --- resources:3.3.1:testResources (default-testResources) @ jsontests --- [INFO] skip non existing resourceDirectory /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/jsonunit/jsontests/src/test/resources [INFO] [INFO] --- compiler:3.13.0:testCompile (default-testCompile) @ jsontests --- [INFO] Recompiling the module because of changed dependency. [INFO] Compiling 2 source files with javac [debug target 8] to target /test-classes [WARNING] bootstrap class path not set in conjunction with - source 8 [INFO] [INFO] --- surefire:3.2.5: test (default- test ) @ jsontests --- [INFO] Using auto detected provider org.apache.maven.surefire.junitplatform.JUnitPlatformProvider [INFO] [INFO] ------------------------------------------------------- [INFO] T E S T S [INFO] ------------------------------------------------------- [INFO] Running org.javacodegeeks.jsonunit.JsonAssertionsUnitTest [INFO] Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.218 s -- in org.javacodegeeks.jsonunit.JsonAssertionsUnitTest [INFO] [INFO] Results: [INFO] [WARNING] Tests run: 1, Failures: 0, Errors: 0, Skipped: 0 [INFO] [INFO] [INFO] --- jar:3.4.1:jar (default-jar) @ jsontests --- [INFO] Building jar: /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/jsonunit/jsontests/target/jsontests-0 .1-SNAPSHOT.jar [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time : 2.907 s [INFO] Finished at: 2025-01-22T22:28:10+05:30 [INFO] ------------------------------------------------------------------------ bhagvanarch@Bhagvans-MacBook-Air jsontests % mvn test [INFO] Scanning for projects... [INFO] gitflow-incremental-builder is disabled. [INFO] [INFO] ----------------------------------------- [INFO] Building jsontests 0.1-SNAPSHOT [INFO] from pom.xml [INFO] --------------------------------[ jar ]--------------------------------- [WARNING] Parameter 'sourceEncoding' is unknown for plugin 'maven-pmd-plugin:3.26.0:check (default)' [WARNING] Parameter 'sourceEncoding' is unknown for plugin 'maven-pmd-plugin:3.26.0:pmd (pmd)' [INFO] [INFO] --- directory:1.0:directory-of (directories) @ jsontests --- [INFO] Directory of org.javacodegeeks:parent-modules set to: /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest [INFO] [INFO] --- install :3.1.2: install - file ( install -jar-lib) @ jsontests --- [INFO] Installing /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/custom-pmd-0 .0.1.jar to /Users/bhagvanarch/ .m2 /repository/org/baeldung/pmd/custom-pmd/0 .0.1 /custom-pmd-0 .0.1.jar [INFO] Installing /var/folders/jm/7m3hf4f1639_vpyg29093b2h0000gn/T/mvninstall16550186167604768808 .pom to /Users/bhagvanarch/ .m2 /repository/org/baeldung/pmd/custom-pmd/0 .0.1 /custom-pmd-0 .0.1.pom [INFO] [INFO] --- resources:3.3.1:resources (default-resources) @ jsontests --- [INFO] skip non existing resourceDirectory /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/jsonunit/jsontests/src/main/resources [INFO] [INFO] --- compiler:3.13.0:compile (default-compile) @ jsontests --- [INFO] Nothing to compile - all classes are up to date . [INFO] [INFO] >>> pmd:3.26.0:check (default) > :pmd @ jsontests >>> [INFO] [INFO] --- pmd:3.26.0:pmd (pmd) @ jsontests --- [WARNING] Parameter 'aggregate' (user property 'aggregate' ) is deprecated: since 3.15.0 Use the goals pmd:aggregate-pmd and pmd:aggregate-cpd instead. See FAQ: Why do I get sometimes false positive and /or false negative violations? for an explanation. [INFO] PMD version: 7.7.0 [INFO] Rendering content with org.apache.maven.skins:maven-fluido-skin:jar:2.0.0-M9 skin [INFO] [INFO] <<< pmd:3.26.0:check (default) < :pmd @ jsontests <<< [INFO] [INFO] [INFO] --- pmd:3.26.0:check (default) @ jsontests --- [WARNING] Parameter 'aggregate' (user property 'aggregate' ) is deprecated: since 3.15.0 Use the goal pmd:aggregate-check or pmd:aggregate-cpd-check instead. [INFO] [INFO] --- resources:3.3.1:testResources (default-testResources) @ jsontests --- [INFO] skip non existing resourceDirectory /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/jsonunit/jsontests/src/test/resources [INFO] [INFO] --- compiler:3.13.0:testCompile (default-testCompile) @ jsontests --- [INFO] Nothing to compile - all classes are up to date . [INFO] [INFO] --- surefire:3.2.5: test (default- test ) @ jsontests --- [INFO] Using auto detected provider org.apache.maven.surefire.junitplatform.JUnitPlatformProvider [INFO] [INFO] ------------------------------------------------------- [INFO] T E S T S [INFO] ------------------------------------------------------- [INFO] Running org.javacodegeeks.jsonunit.JsonAssertionsUnitTest [INFO] Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.198 s -- in org.javacodegeeks.jsonunit.JsonAssertionsUnitTest [INFO] [INFO] Results: [INFO] [WARNING] Tests run: 1, Failures: 0, Errors: 0, Skipped: 0 [INFO] [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time : 2.095 s [INFO] Finished at: 2025-01-22T22:28:17+05:30 [INFO] ------------------------------------------------------------------------ bhagvanarch@Bhagvans-MacBook-Air jsontests % |
Now let us look at the supporting features in JsonUnit.
3. Supporting Features of JsonUnit
JsonUnit is packed with various features to make JSON testing more efficient and flexible.
JsonUnit offers advanced features like partial matching, ignoring specific fields, and custom comparison strategies. As you become more comfortable with the basics, explore these advanced capabilities to enhance your tests.
Here’s an overview of some key features that enhance its functionality:
- Partial Matching
JsonUnit allows you to perform partial matches on JSON structures, which is particularly useful when only a subset of fields needs to be verified. This feature ensures that tests remain relevant even when the JSON structure evolves. - Ignoring Fields
You can instruct JsonUnit to ignore specific fields during comparisons, such as timestamps or unique identifiers, which might change with every request. This helps focus on the essential parts of the JSON data without being affected by variable elements. - Custom Comparison Strategies
JsonUnit supports custom comparison strategies, enabling you to define how certain fields should be compared. This is useful for handling fields with complex data types or custom validation logic. - Path Assertions
With JsonUnit, you can make assertions on specific JSON paths, allowing precise verification of nested elements within large JSON structures. This feature provides granular control over which parts of the JSON data are tested. - Tolerance for Numeric Comparisons
JsonUnit offers tolerance for numeric comparisons, making it possible to assert that numeric values fall within an acceptable range. This is especially beneficial when dealing with floating-point calculations with minor variations. - Integration with Testing Frameworks
JsonUnit integrates seamlessly with popular testing frameworks like JUnit and TestNG, making it easy to incorporate JSON assertions into your existing test suite. This integration ensures consistency and efficiency in your testing process. - Detailed Error Messages
When a test fails, JsonUnit provides detailed error messages that pinpoint the exact discrepancies between the expected and actual JSON data. This makes debugging easier and faster, as you can quickly identify and address issues. - Schema Validation
JsonUnit can be used in conjunction with JSON schema validation libraries to ensure that your JSON data conforms to predefined schemas. This adds an extra layer of validation to your tests, ensuring data integrity and consistency.
By leveraging these features, JsonUnit offers a comprehensive toolkit for robust and flexible JSON testing, helping developers maintain high-quality applications with reliable data interchange.
Let us look at the Java code for the implementation of the supporting features:
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 083 084 085 086 087 088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 103 104 105 106 107 108 109 110 111 112 113 | package com.baeldung.jsonunit; import static net.javacrumbs.jsonunit.assertj.JsonAssertions.assertThatJson; import static net.javacrumbs.jsonunit.assertj.JsonAssertions.json; import java.util.List; import org.junit.jupiter.api.Test; import net.javacrumbs.jsonunit.core.Option; class JsonUnitAssertionsUnitTest { @Test void whenWeVerifyAJsonObject_thenItContainsKeyValueEntries() { String articleJson = "" " { "name" : "A Guide to Spring Boot" , "tags" : [ "java" , "spring boot" , "backend" ] } "" "; assertThatJson(articleJson).isObject() .containsEntry( "name" , "A Guide to Spring Boot" ) .containsEntry( "tags" , List.of( "java" , "spring boot" , "backend" )); } @Test void whenWeVerifyAJsonObject_thenItMatchesAnExpectedJsonString() { String articleJson = "" " { "name" : "A Guide to Spring Boot" , "tags" : [ "java" , "spring boot" , "backend" ] } "" "; assertThatJson(articleJson).isObject() .isEqualTo(json( "" " { "name" : "A Guide to Spring Boot" , "tags" : [ "java" , "spring boot" , "backend" ] } "" ")); } @Test void whenWeVerifyAJsonArrayField_thenItContainsExpectedValues() { String articleJson = "" " { "name" : "A Guide to Spring Boot" , "tags" : [ "java" , "spring boot" , "backend" ] } "" "; assertThatJson(articleJson).isObject() .containsEntry( "name" , "A Guide to Spring Boot" ) .node( "tags" ) .isArray() .containsExactlyInAnyOrder( "java" , "spring boot" , "backend" ); } @Test void whenWeVerifyTheListOfTags_thenItContainsExpectedStrings() { String articleJson = "" " { "name" : "A Guide to Spring Boot" , "tags" : [ "java" , "spring boot" , "backend" ] } "" "; assertThatJson(articleJson).when(Option.IGNORING_ARRAY_ORDER) .when(Option.IGNORING_EXTRA_ARRAY_ITEMS) .node( "tags" ) .isEqualTo(json( "" " [ "backend" , "java" ] "" ")); } @Test void whenWeVerifyJsonObject_thenItMatchesAgainstPlaceholders() { String articleJson = "" " { "name" : "A Guide to Spring Boot" , "tags" : [ "java" , "spring boot" , "backend" ] } "" "; assertThatJson(articleJson).isEqualTo(json( "" " { "name" : "${json-unit.any-string}" , "tags" : "${json-unit.ignore-element}" } "" ")); } @Test void whenWeVerifyJsonObjectIgnoringTags_thenItEqualsTheExpectedJson() { String articleJson = "" " { "name" : "A Guide to Spring Boot" , "tags" : [ "java" , "spring boot" , "backend" ] } "" "; assertThatJson(articleJson).whenIgnoringPaths( "tags" ) .isEqualTo(json( "" " { "name" : "A Guide to Spring Boot" , "tags" : [ "ignored" , "tags" ] } "" ")); } } |
You can compile and execute the above code with the following commands:
1 2 | mvn package mvn test |
The output for the above commands when executed is shown below:
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 083 084 085 086 087 088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 | bhagvanarch@Bhagvans-MacBook-Air jsontests % mvn package [INFO] Scanning for projects... [INFO] gitflow-incremental-builder is disabled. [INFO] [INFO] ----------------------------------------- [INFO] Building jsontests 0.1-SNAPSHOT [INFO] from pom.xml [INFO] --------------------------------[ jar ]--------------------------------- [WARNING] Parameter 'sourceEncoding' is unknown for plugin 'maven-pmd-plugin:3.26.0:check (default)' [WARNING] Parameter 'sourceEncoding' is unknown for plugin 'maven-pmd-plugin:3.26.0:pmd (pmd)' [INFO] [INFO] --- directory:1.0:directory-of (directories) @ jsontests --- [INFO] Directory of org.javacodegeeks:parent-modules set to: /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest [INFO] [INFO] --- install :3.1.2: install - file ( install -jar-lib) @ jsontests --- [INFO] Installing /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/custom-pmd-0 .0.1.jar to /Users/bhagvanarch/ .m2 /repository/org/baeldung/pmd/custom-pmd/0 .0.1 /custom-pmd-0 .0.1.jar [INFO] Installing /var/folders/jm/7m3hf4f1639_vpyg29093b2h0000gn/T/mvninstall17870915735783281865 .pom to /Users/bhagvanarch/ .m2 /repository/org/baeldung/pmd/custom-pmd/0 .0.1 /custom-pmd-0 .0.1.pom [INFO] [INFO] --- resources:3.3.1:resources (default-resources) @ jsontests --- [INFO] skip non existing resourceDirectory /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/jsonunit/jsontests/src/main/resources [INFO] [INFO] --- compiler:3.13.0:compile (default-compile) @ jsontests --- [INFO] Recompiling the module because of changed source code. [INFO] Compiling 3 source files with javac [debug target 8] to target /classes [WARNING] bootstrap class path not set in conjunction with - source 8 [INFO] [INFO] >>> pmd:3.26.0:check (default) > :pmd @ jsontests >>> [INFO] [INFO] --- pmd:3.26.0:pmd (pmd) @ jsontests --- [WARNING] Parameter 'aggregate' (user property 'aggregate' ) is deprecated: since 3.15.0 Use the goals pmd:aggregate-pmd and pmd:aggregate-cpd instead. See FAQ: Why do I get sometimes false positive and /or false negative violations? for an explanation. [INFO] PMD version: 7.7.0 [INFO] Rendering content with org.apache.maven.skins:maven-fluido-skin:jar:2.0.0-M9 skin [INFO] [INFO] <<< pmd:3.26.0:check (default) < :pmd @ jsontests <<< [INFO] [INFO] [INFO] --- pmd:3.26.0:check (default) @ jsontests --- [WARNING] Parameter 'aggregate' (user property 'aggregate' ) is deprecated: since 3.15.0 Use the goal pmd:aggregate-check or pmd:aggregate-cpd-check instead. [INFO] [INFO] --- resources:3.3.1:testResources (default-testResources) @ jsontests --- [INFO] skip non existing resourceDirectory /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/jsonunit/jsontests/src/test/resources [INFO] [INFO] --- compiler:3.13.0:testCompile (default-testCompile) @ jsontests --- [INFO] Recompiling the module because of changed dependency. [INFO] Compiling 2 source files with javac [debug target 8] to target /test-classes [WARNING] bootstrap class path not set in conjunction with - source 8 [INFO] [INFO] --- surefire:3.2.5: test (default- test ) @ jsontests --- [INFO] Using auto detected provider org.apache.maven.surefire.junitplatform.JUnitPlatformProvider [INFO] [INFO] ------------------------------------------------------- [INFO] T E S T S [INFO] ------------------------------------------------------- [INFO] Running org.javacodegeeks.jsonunit.JsonAssertionsUnitTest [INFO] Tests run: 6, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.218 s -- in org.javacodegeeks.jsonunit.JsonAssertionsUnitTest [INFO] [INFO] Results: [INFO] [WARNING] Tests run: 6, Failures: 0, Errors: 0, Skipped: 0 [INFO] [INFO] [INFO] --- jar:3.4.1:jar (default-jar) @ jsontests --- [INFO] Building jar: /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/jsonunit/jsontests/target/jsontests-0 .1-SNAPSHOT.jar [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time : 2.907 s [INFO] Finished at: 2025-01-22T22:28:10+05:30 [INFO] ------------------------------------------------------------------------ bhagvanarch@Bhagvans-MacBook-Air jsontests % mvn test [INFO] Scanning for projects... [INFO] gitflow-incremental-builder is disabled. [INFO] [INFO] ----------------------------------------- [INFO] Building jsontests 0.1-SNAPSHOT [INFO] from pom.xml [INFO] --------------------------------[ jar ]--------------------------------- [WARNING] Parameter 'sourceEncoding' is unknown for plugin 'maven-pmd-plugin:3.26.0:check (default)' [WARNING] Parameter 'sourceEncoding' is unknown for plugin 'maven-pmd-plugin:3.26.0:pmd (pmd)' [INFO] [INFO] --- directory:1.0:directory-of (directories) @ jsontests --- [INFO] Directory of org.javacodegeeks:parent-modules set to: /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest [INFO] [INFO] --- install :3.1.2: install - file ( install -jar-lib) @ jsontests --- [INFO] Installing /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/custom-pmd-0 .0.1.jar to /Users/bhagvanarch/ .m2 /repository/org/baeldung/pmd/custom-pmd/0 .0.1 /custom-pmd-0 .0.1.jar [INFO] Installing /var/folders/jm/7m3hf4f1639_vpyg29093b2h0000gn/T/mvninstall16550186167604768808 .pom to /Users/bhagvanarch/ .m2 /repository/org/baeldung/pmd/custom-pmd/0 .0.1 /custom-pmd-0 .0.1.pom [INFO] [INFO] --- resources:3.3.1:resources (default-resources) @ jsontests --- [INFO] skip non existing resourceDirectory /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/jsonunit/jsontests/src/main/resources [INFO] [INFO] --- compiler:3.13.0:compile (default-compile) @ jsontests --- [INFO] Nothing to compile - all classes are up to date . [INFO] [INFO] >>> pmd:3.26.0:check (default) > :pmd @ jsontests >>> [INFO] [INFO] --- pmd:3.26.0:pmd (pmd) @ jsontests --- [WARNING] Parameter 'aggregate' (user property 'aggregate' ) is deprecated: since 3.15.0 Use the goals pmd:aggregate-pmd and pmd:aggregate-cpd instead. See FAQ: Why do I get sometimes false positive and /or false negative violations? for an explanation. [INFO] PMD version: 7.7.0 [INFO] Rendering content with org.apache.maven.skins:maven-fluido-skin:jar:2.0.0-M9 skin [INFO] [INFO] <<< pmd:3.26.0:check (default) < :pmd @ jsontests <<< [INFO] [INFO] [INFO] --- pmd:3.26.0:check (default) @ jsontests --- [WARNING] Parameter 'aggregate' (user property 'aggregate' ) is deprecated: since 3.15.0 Use the goal pmd:aggregate-check or pmd:aggregate-cpd-check instead. [INFO] [INFO] --- resources:3.3.1:testResources (default-testResources) @ jsontests --- [INFO] skip non existing resourceDirectory /Users/bhagvanarch/kazdesk/javacodegeeks/code/jsonunit_jan_21/jsonunittest/jsonunit/jsontests/src/test/resources [INFO] [INFO] --- compiler:3.13.0:testCompile (default-testCompile) @ jsontests --- [INFO] Nothing to compile - all classes are up to date . [INFO] [INFO] --- surefire:3.2.5: test (default- test ) @ jsontests --- [INFO] Using auto detected provider org.apache.maven.surefire.junitplatform.JUnitPlatformProvider [INFO] [INFO] ------------------------------------------------------- [INFO] T E S T S [INFO] ------------------------------------------------------- [INFO] Running org.javacodegeeks.jsonunit.JsonAssertionsUnitTest [INFO] Tests run: 6, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.198 s -- in org.javacodegeeks.jsonunit.JsonAssertionsUnitTest [INFO] [INFO] Results: [INFO] [WARNING] Tests run: 6, Failures: 0, Errors: 0, Skipped: 0 [INFO] [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time : 2.095 s [INFO] Finished at: 2025-01-22T22:28:17+05:30 [INFO] ------------------------------------------------------------------------ bhagvanarch@Bhagvans-MacBook-Air jsontests % |
4. Conclusion
In this tutorial, we’ve explored the essential aspects of using JsonUnit for JSON unit test assertions. From getting started with adding JsonUnit to your project, to writing basic and advanced tests, we covered various features and techniques to enhance your JSON testing practices. By leveraging JsonUnit’s powerful capabilities, such as partial matching, ignoring fields, custom comparison strategies, and path assertions, you can ensure that your APIs deliver consistent and accurate responses.
Integrating JsonUnit with popular testing frameworks and utilizing its detailed error messages will help streamline your testing process and improve debugging efficiency. With these tools at your disposal, you’re well-equipped to maintain high-quality applications with reliable data interchange.
Happy testing, and may your JSON assertions always be accurate and reliable!
5. Download
You can download the full source code of this example here: JSON Unit Test Assertions Using JsonUnit